游戏展示
游戏开发
创建 BomberMan 游戏
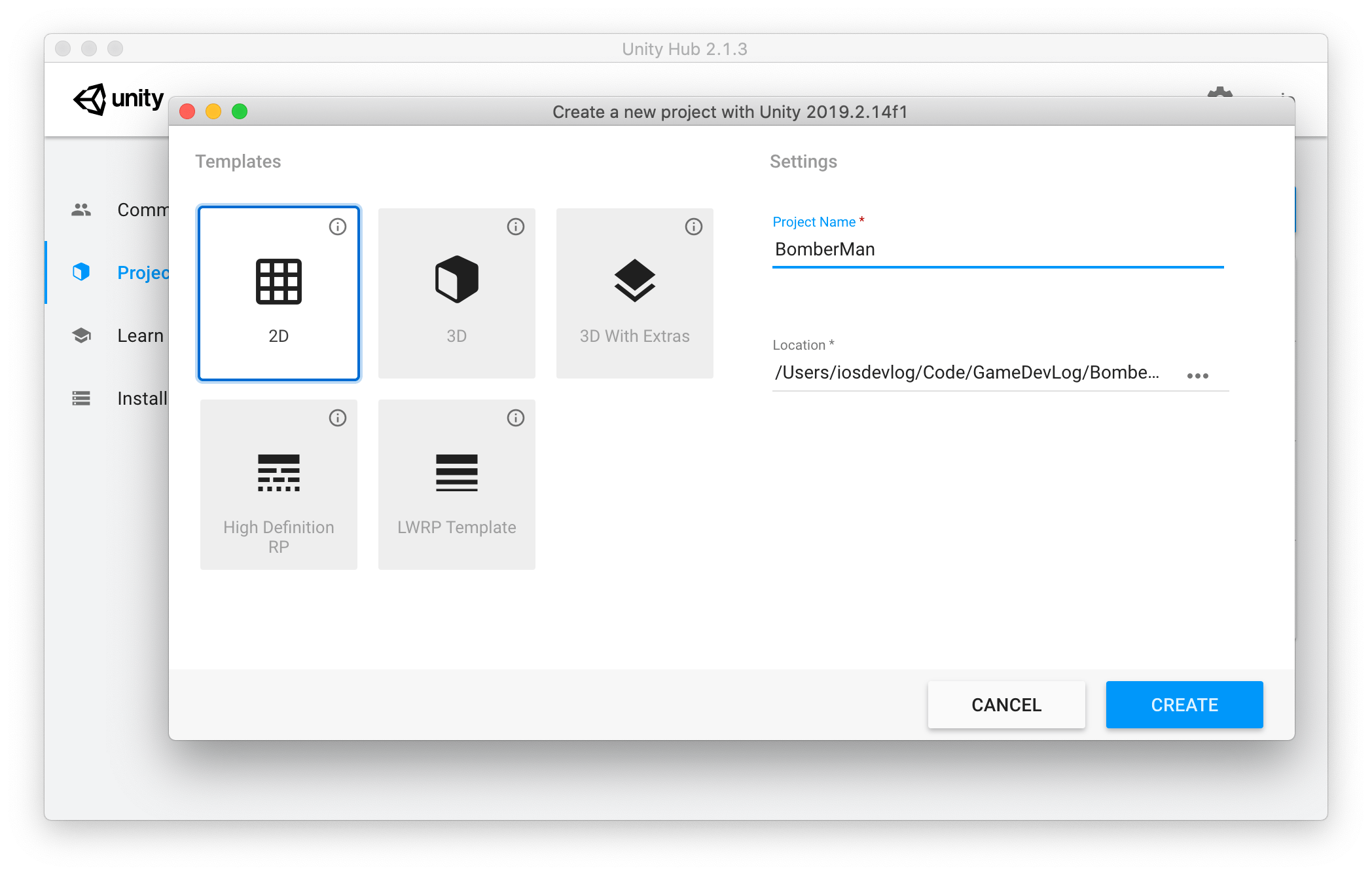
导入资源
可以从 GitHub 上面查看到。
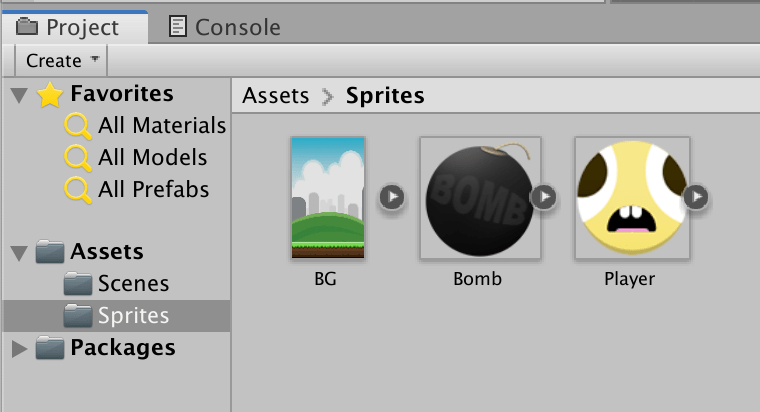
设置分辨率 400x800
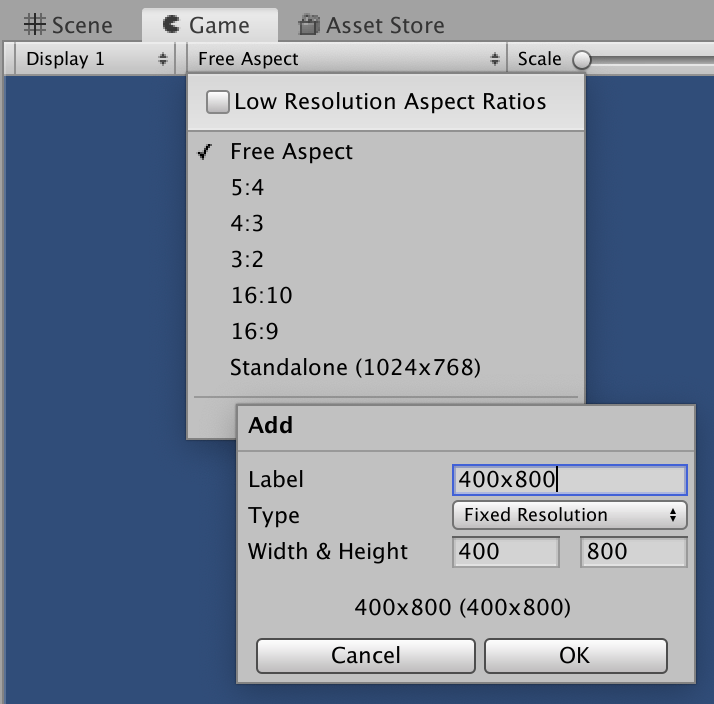
从 Project > Sprites
中拖动 BG
到 GamePlay
Scene。
添加背景
- BG
- Transform
- Scale
- X:
1.5
- Y:
1.5
- X:
- Scale
- Transform
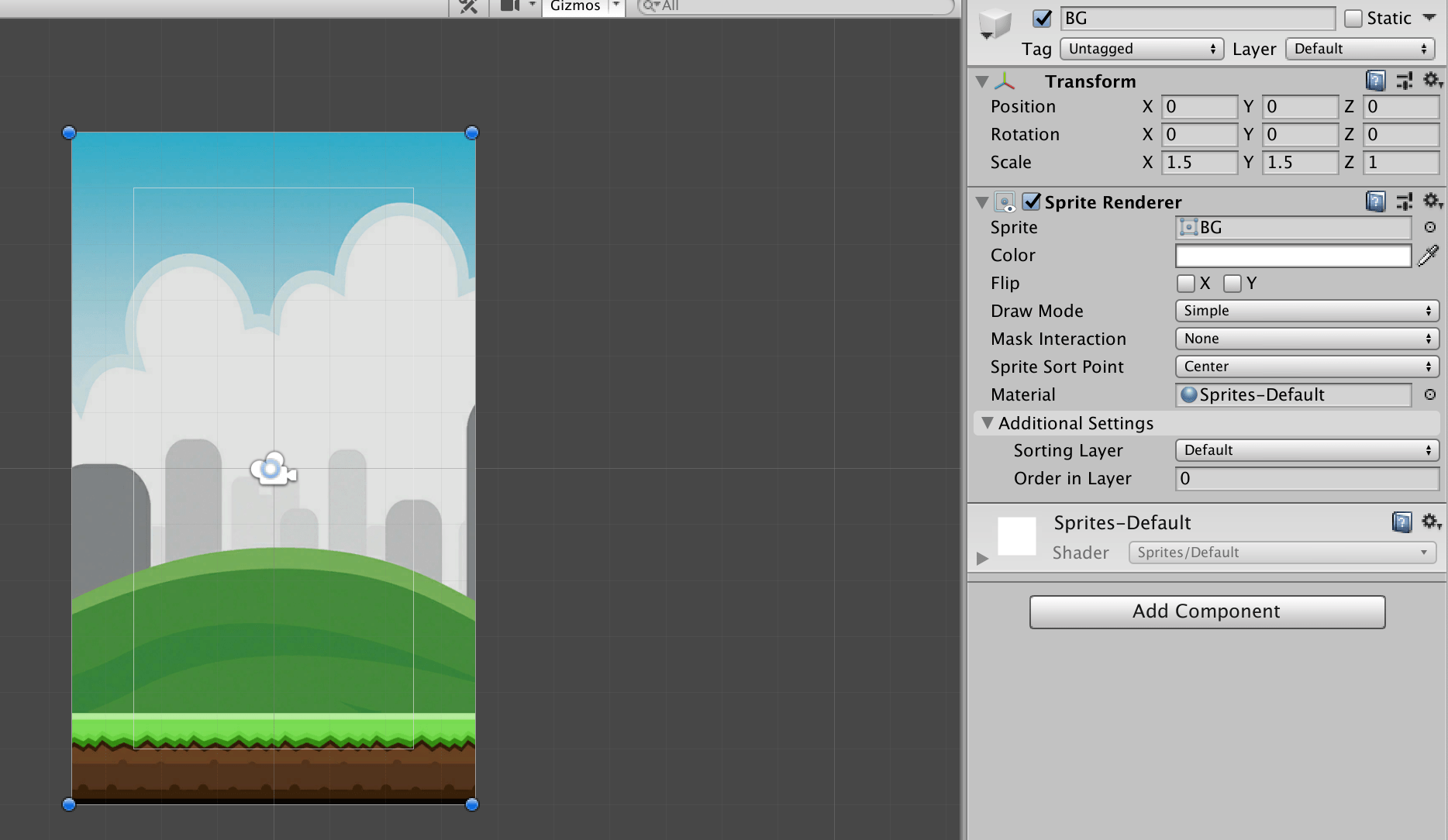
添加 Player
从 Project > Sprites
中拖动 Player
到 GamePlay
Scene。
- Player
- Transform
- Position
- X:
0
- Y:
-3.66
- X:
- Position
- Sprite Renderer
- Additional Settings
- Order in Layer:
1
- Order in Layer:
- Additional Settings
- Transform
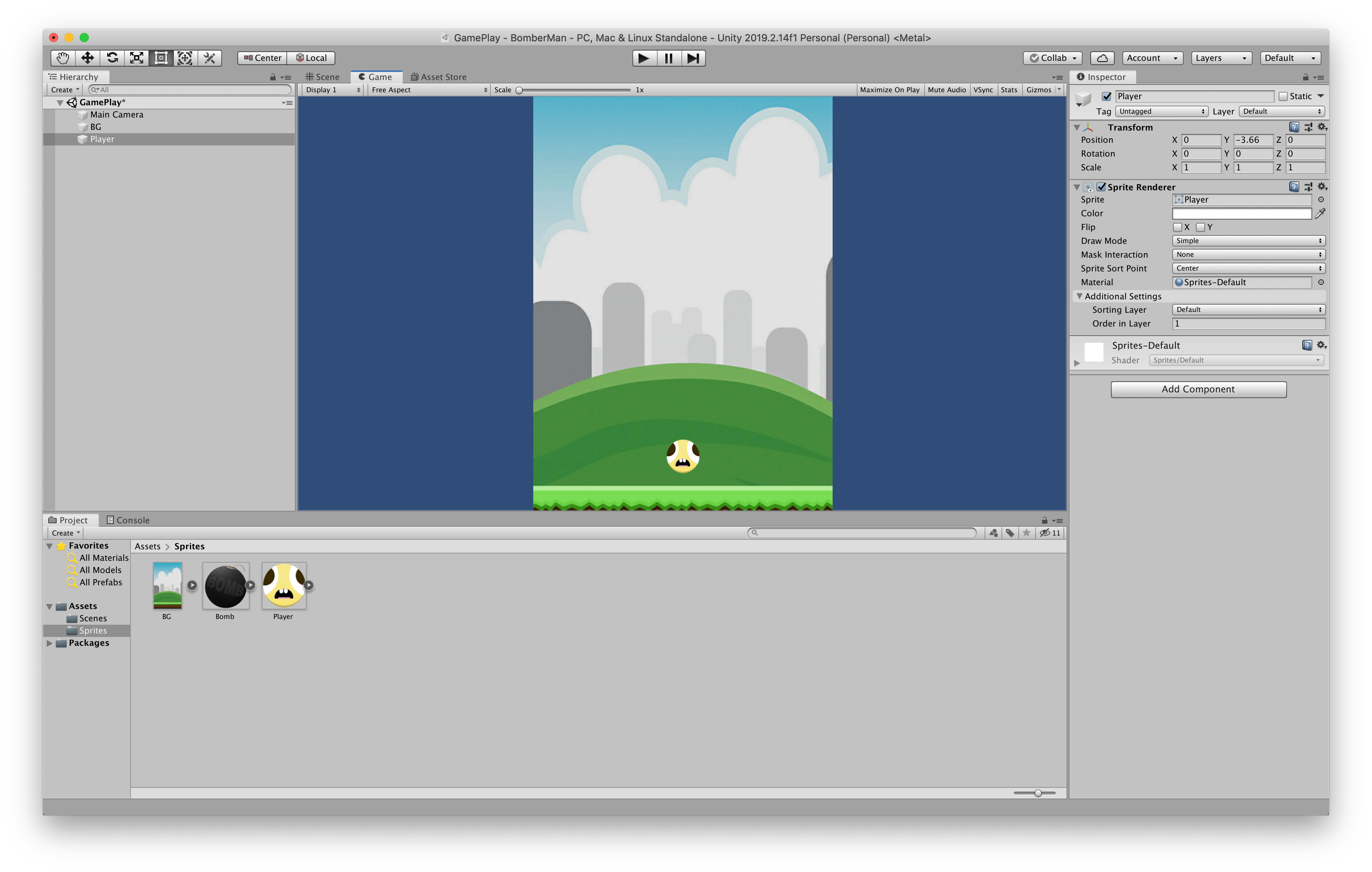
给 Player 添加 Rigibody 2D
- Player
- Add Component
- Rigibody 2D
- Add Component
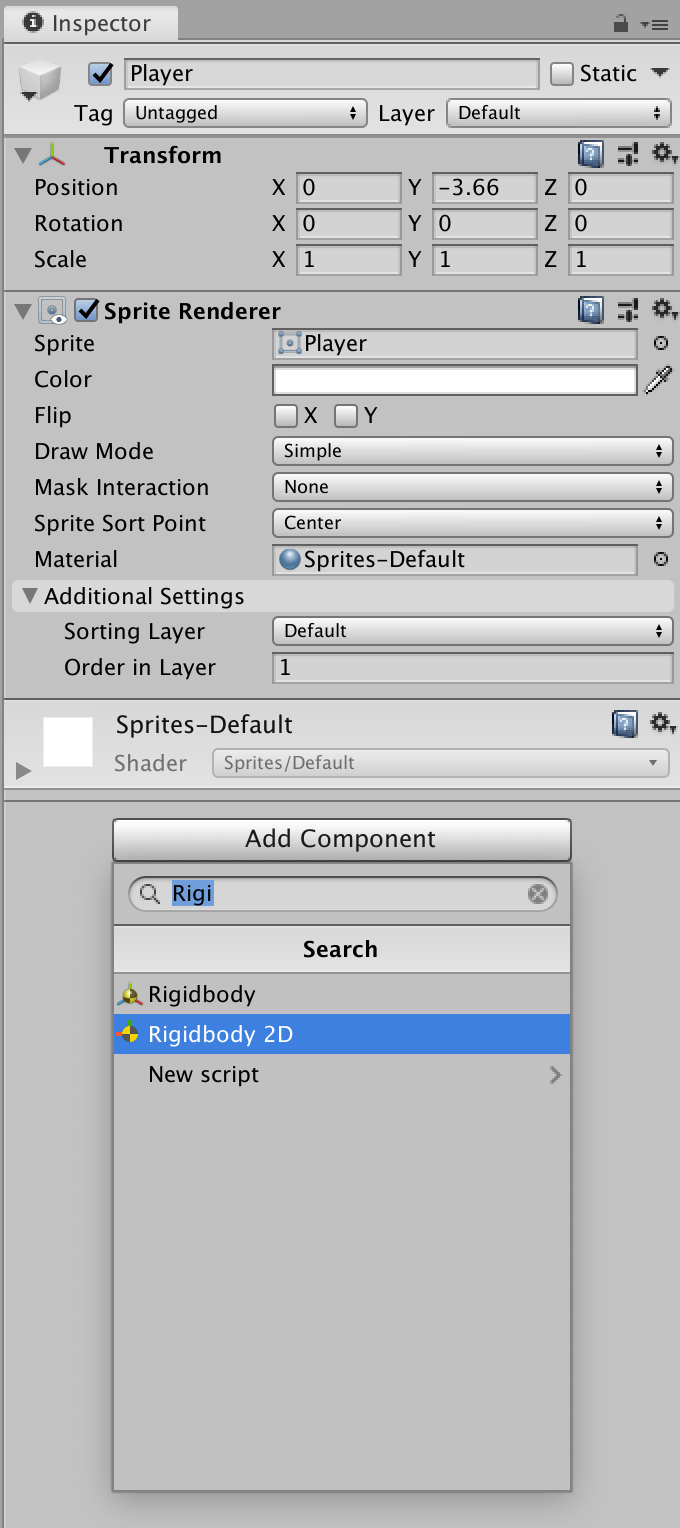
Kinematic
: 运动学
如果该属性设置为 true
表示该物体运动状态不受外力,碰撞和关节的影响,而只受到动画以及附加在物体上的脚本影响,但是该物体仍然能改变其他物体运动状态
- Player
- Rigibody 2D
- Body Type:
Kinematic
- Body Type:
- Rigibody 2D
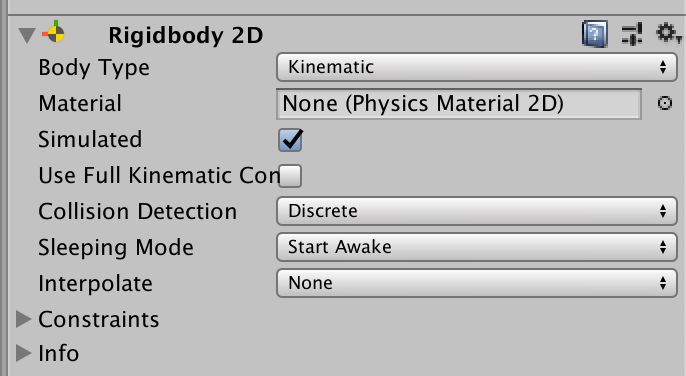
给 Player 添加 Circle Collider 2D
- Player
- Circle Collider 2D
- Is Trigger:
True
- Radius:
0.41
- Is Trigger:
- Circle Collider 2D
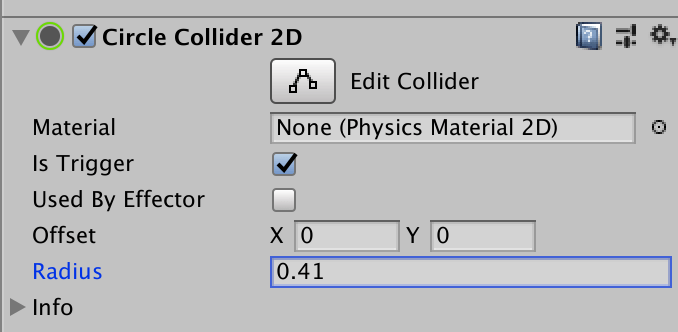
创建 PlayerScripts
- Project
- Assets
- Create
- Folder:
Scripts
- Folder:
- Scripts
- Create
- C# Script:
PlayerScript
- Create
- Assets
添加 PlayerScript
到 Player
组件上。
1 | using System.Collections; |
2 | using System.Collections.Generic; |
3 | using UnityEngine; |
4 | |
5 | public class PlayerScript : MonoBehaviour |
6 | { |
7 | public float speed = 10f; |
8 | private float minX = -2.55f; |
9 | private float maxX = 2.55f; |
10 | |
11 | // Update is called once per frame |
12 | void Update() |
13 | { |
14 | MovePlayer(); |
15 | } |
16 | |
17 | void MovePlayer() |
18 | { |
19 | float h = Input.GetAxis("Horizontal"); |
20 | Vector2 currentPosition = transform.position; |
21 | |
22 | if (h > 0) |
23 | { |
24 | // going to the right side |
25 | currentPosition.x += speed * Time.deltaTime; |
26 | } |
27 | else if (h < 0) |
28 | { |
29 | // going to the left side |
30 | currentPosition.x -= speed * Time.deltaTime; |
31 | } |
32 | |
33 | if (currentPosition.x < minX) |
34 | { |
35 | currentPosition.x = minX; |
36 | } |
37 | else if (currentPosition.x > maxX) |
38 | { |
39 | currentPosition.x = maxX; |
40 | } |
41 | |
42 | transform.position = currentPosition; |
43 | } |
44 | } |
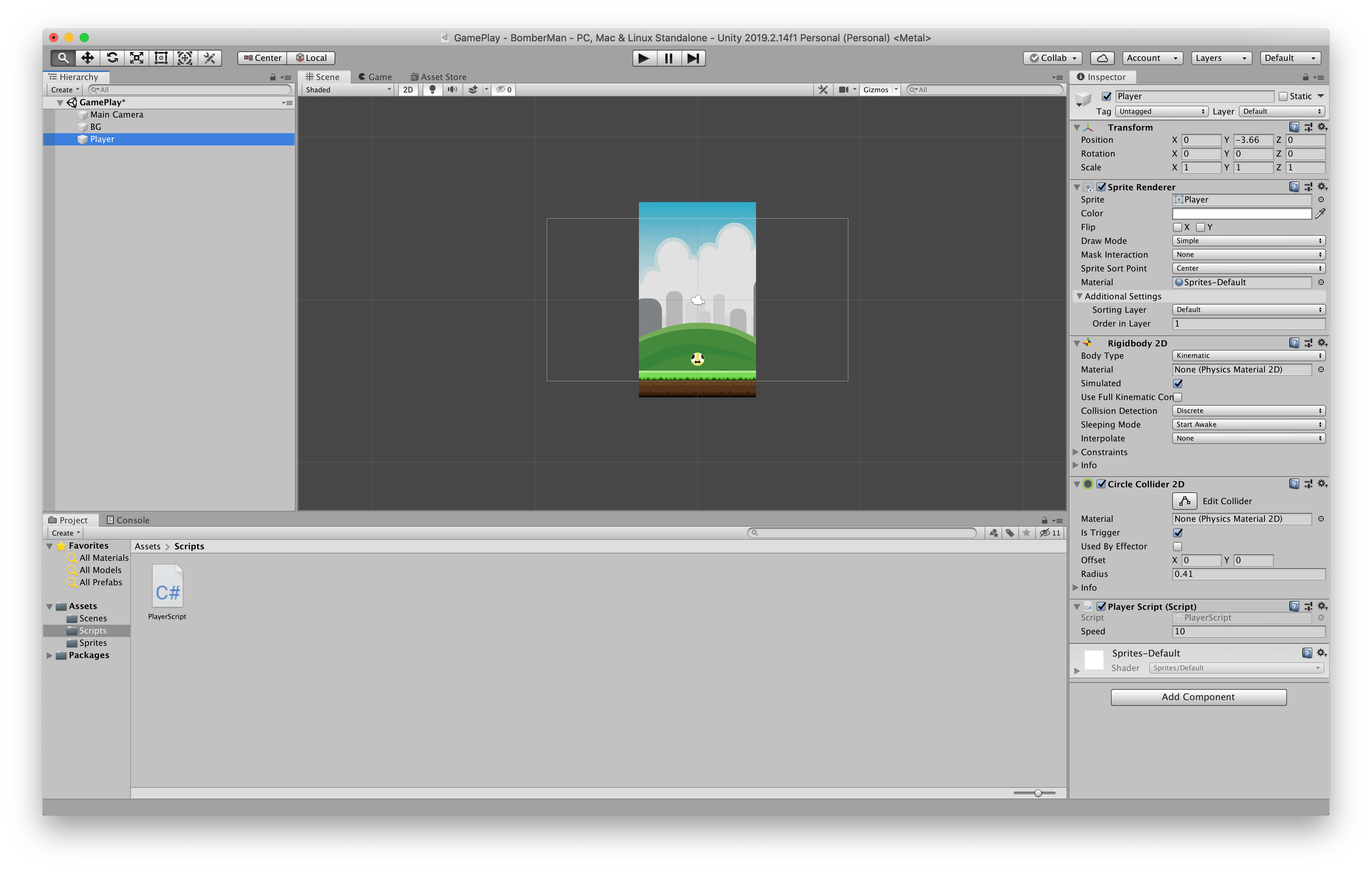
运行游戏,使用 AWDS
中的 AD 或者使用 左右方向键
移动
添加 Bomb
- 从
Project > Sprites
中拖动Bomb
到GamePlay
Scene。 - 添加
Tag
- 添加
Rigibody 2D
- 添加
Circle Collider 2D
- Bomb
- Tag:
Bomb
- Transform
- Position
- X:
0
- Y:
3.28
- X:
- Scale
- X:
0.8
- Y:
0.8
- X:
- Position
- Rigibody 2D
- Circle Collider 2D
- Is Trigger:
True
- Is Trigger:
- Tag:
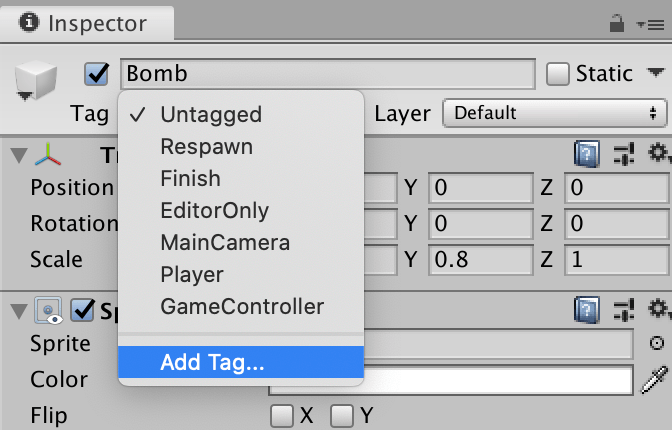
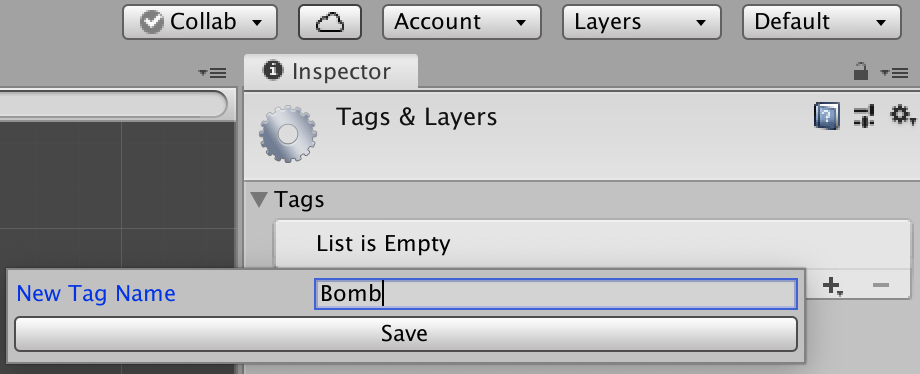
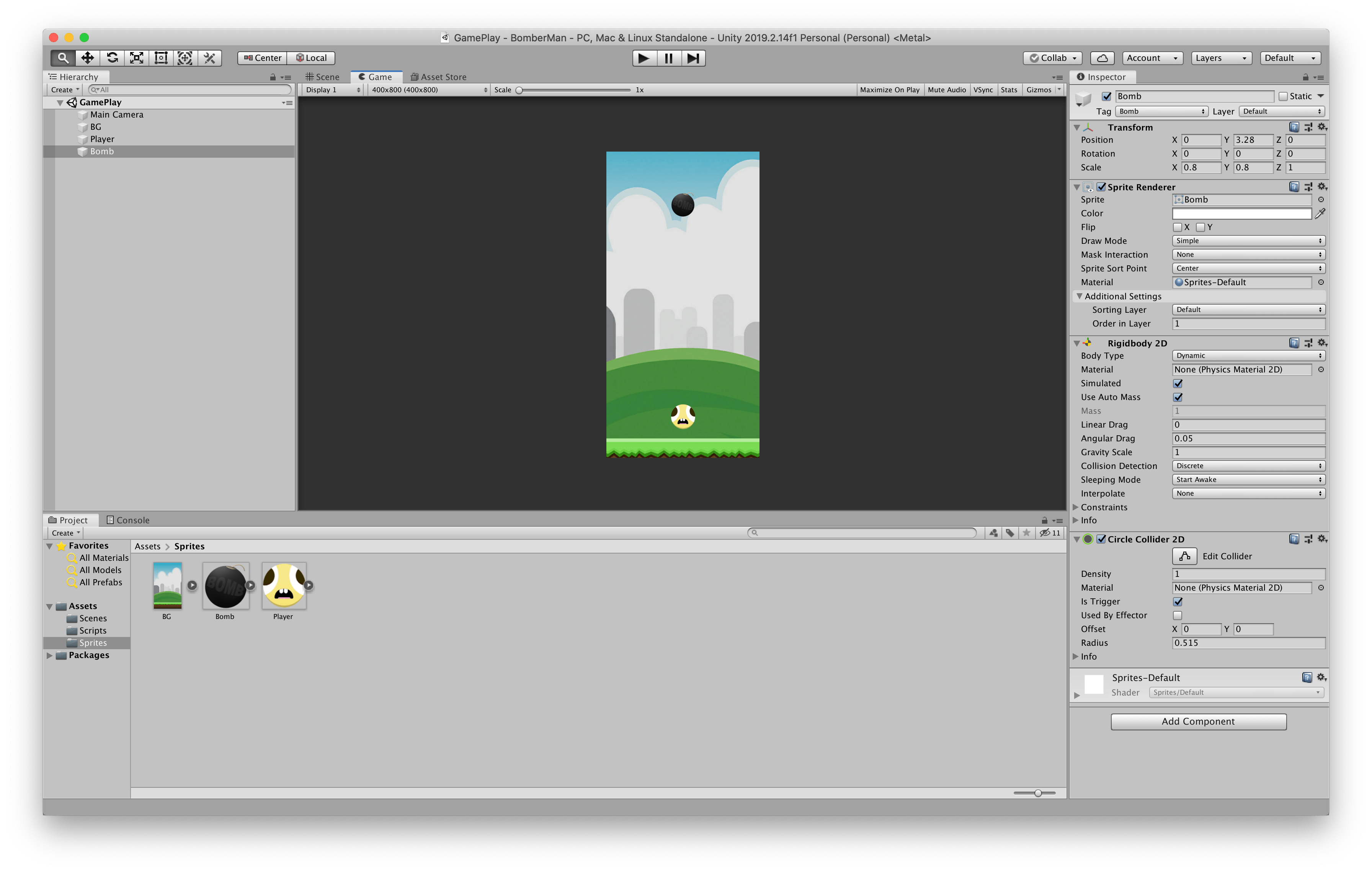
Prefabs / 预设体
- Project
- Assets
- Create
- Folders:
Prefabs
- Folders:
- Prefabs
- Create
- Assets
将 Hirearchy > GamePay > Bomb
拖到 Prefabs
中。
删除 Hirearchy > GamePay > Bomb
。
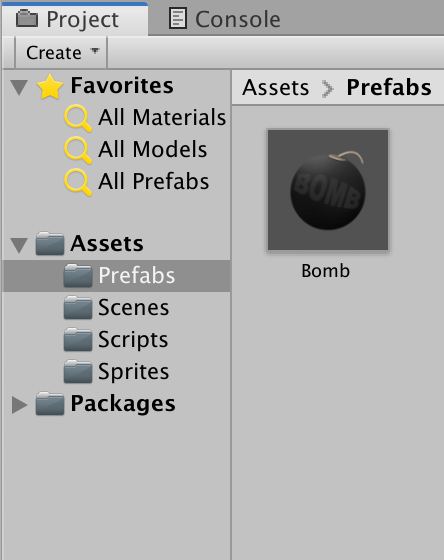
Spawner / 生成器
- Hirearchy
- Create Empty:
Spawner
- Create Empty:
- Inspector
- Spawner
- Select Icon (左上角)
- Transform:
Reset
- Position
- X:
0
- Y:
5.6
- X:
- Position
- Spawner
创建 SpawnerScripts
- Project
- Assets
- Scripts
- Create
- C# Script:
SpawnerScripts
- Scripts
- Assets
添加 SpawnerScripts
到 Spawner
组件上。
1 | using System.Collections; |
2 | using System.Collections.Generic; |
3 | using UnityEngine; |
4 | |
5 | public class SpawnerScript : MonoBehaviour |
6 | { |
7 | public GameObject bombPrefab; |
8 | |
9 | private float minX = -2.55f; |
10 | private float maxX = 2.55f; |
11 | |
12 | void Start() |
13 | { |
14 | StartCoroutine(SpawnBombs()); |
15 | } |
16 | |
17 | IEnumerator SpawnBombs() |
18 | { |
19 | yield return new WaitForSeconds(Random.Range(0f, 1f)); |
20 | |
21 | Instantiate(bombPrefab, new Vector2(Random.Range(minX, maxX), transform.position.y), |
22 | Quaternion.identity); |
23 | |
24 | StartCoroutine(SpawnBombs()); |
25 | |
26 | } |
27 | } |
将 Prefabs > Bomb
拖动到 Hierarchy > Spawner
上。
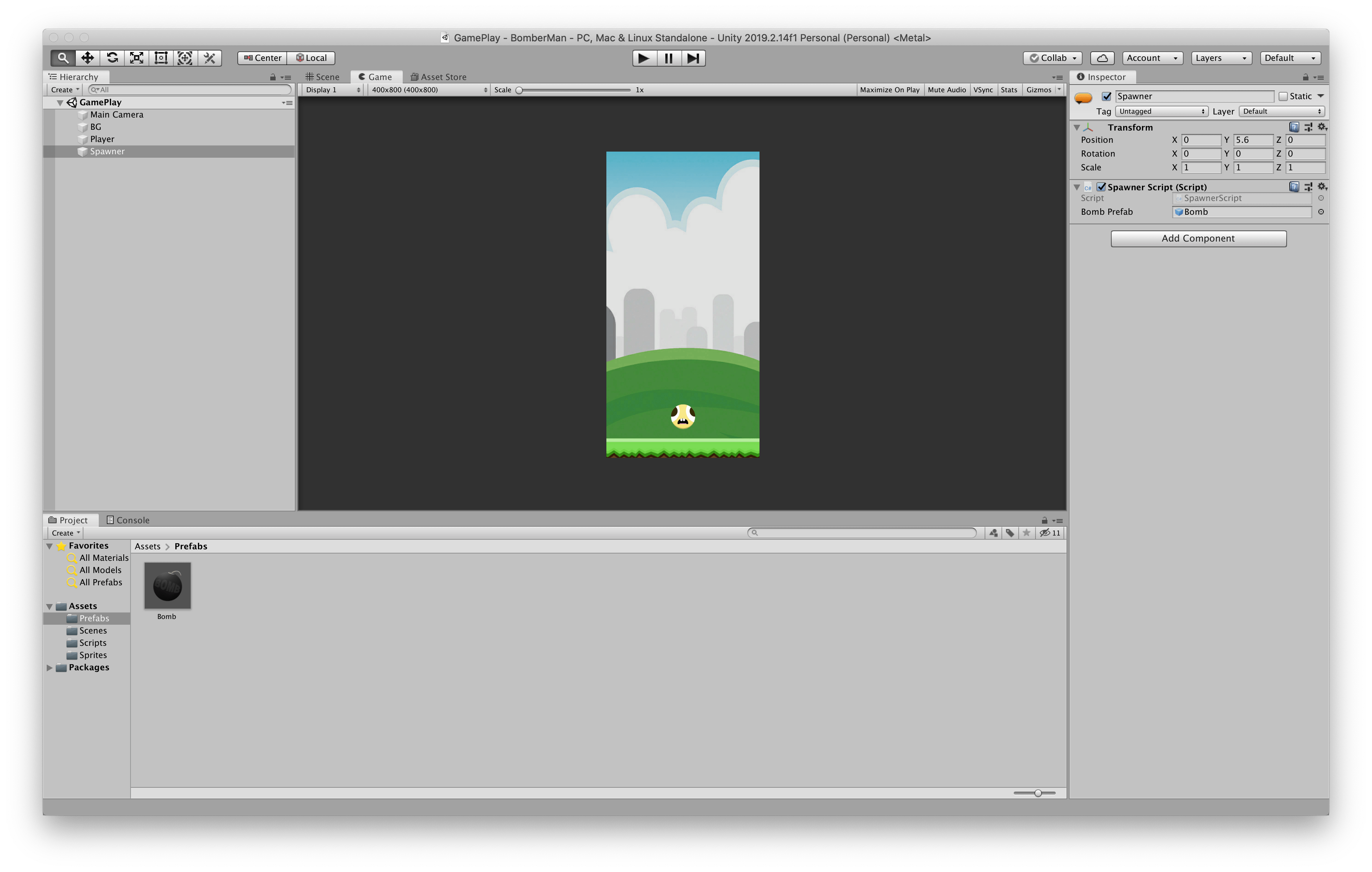
Collector / 收集器
- Hirearchy
- Create Empty:
Collector
- Create Empty:
- Inspector
- Collector
- Select Icon (左上角)
- Transform:
Reset
- Position
- X:
0
- Y:
-6.625
- X:
- Position
- Box Collider 2D
- Is Trigger:
True
- Size
- X:
7.4
- Y:
1
- X:
- Info
- Bounds
- Center
- X: 0
- Y: -4.9
- Center
- Is Trigger:
- Collector
创建 CollectorScripts
Score Text
- Hirearchy
- Create
- UI
- Text
- UI
- Create
- Inspector
- Rect Transform
- Archors
- top
- left
- Pos X:
125.5
- Pos Y:
125.5
- Width:
224
- Height:
50
- Archors
- Text(Script)
- Text:
Score: 0
- Character
- Font Size:
36
- Font Size:
- Paragraph
- V:
Center
- V:
- Color:
White
- Text:
- Rect Transform
- Project
- Assets
- Scripts
- Create
- C# Script:
CollectorScripts
- Scripts
- Assets
添加 CollectorScripts
到 Collector
组件上。
1 | using System.Collections; |
2 | using System.Collections.Generic; |
3 | using UnityEngine; |
4 | using UnityEngine.UI; |
5 | |
6 | public class CollectorScript : MonoBehaviour |
7 | { |
8 | public Text scoreText; |
9 | private int score; |
10 | |
11 | private void OnTriggerEnter2D(Collider2D collision) |
12 | { |
13 | if (collision.tag == "Bomb") |
14 | { |
15 | IncreaseScore(); |
16 | collision.gameObject.SetActive(false); |
17 | } |
18 | } |
19 | |
20 | void IncreaseScore() |
21 | { |
22 | score++; |
23 | |
24 | scoreText.text = "Score: " + score; |
25 | } |
26 | } |
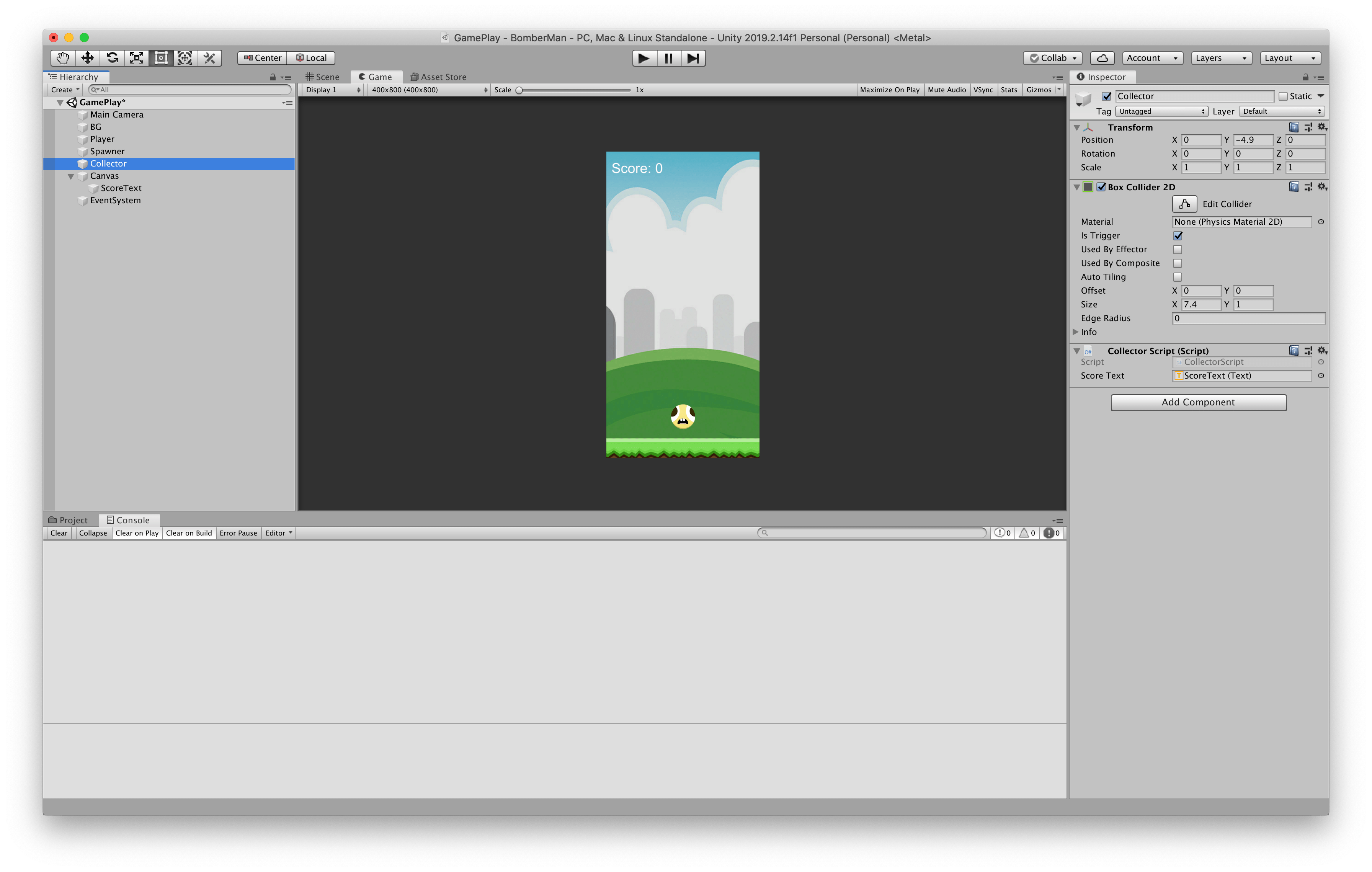
重置游戏
Score Text
:
Inspector
- Rect Transform
- Pos X:
180
- Pos Y:
-60
- Width:
300
- Height:
100
- Pos X:
- Text(Script)
- Text:
High Score: 0\nScore: 0
- Text:
- Rect Transform
修改
CollectorScripts
代码。
1 | using System.Collections; |
2 | using System.Collections.Generic; |
3 | using UnityEngine; |
4 | using UnityEngine.UI; |
5 | |
6 | public class CollectorScript : MonoBehaviour |
7 | { |
8 | public Text scoreText; |
9 | private int score; |
10 | private static int highScore; |
11 | |
12 | private void Start() |
13 | { |
14 | DisplayScore(); |
15 | } |
16 | |
17 | private void OnTriggerEnter2D(Collider2D collision) |
18 | { |
19 | if (collision.tag == "Bomb") |
20 | { |
21 | IncreaseScore(); |
22 | collision.gameObject.SetActive(false); |
23 | } |
24 | } |
25 | |
26 | void DisplayScore() |
27 | { |
28 | scoreText.text = "High Score: " + highScore + "\nScore: " + score; |
29 | } |
30 | |
31 | void IncreaseScore() |
32 | { |
33 | score++; |
34 | |
35 | if (score > highScore) |
36 | { |
37 | highScore = score; |
38 | } |
39 | |
40 | DisplayScore(); |
41 | } |
42 | } |
导出游戏
- File
- Build Settings (Shift + Cmd + B)
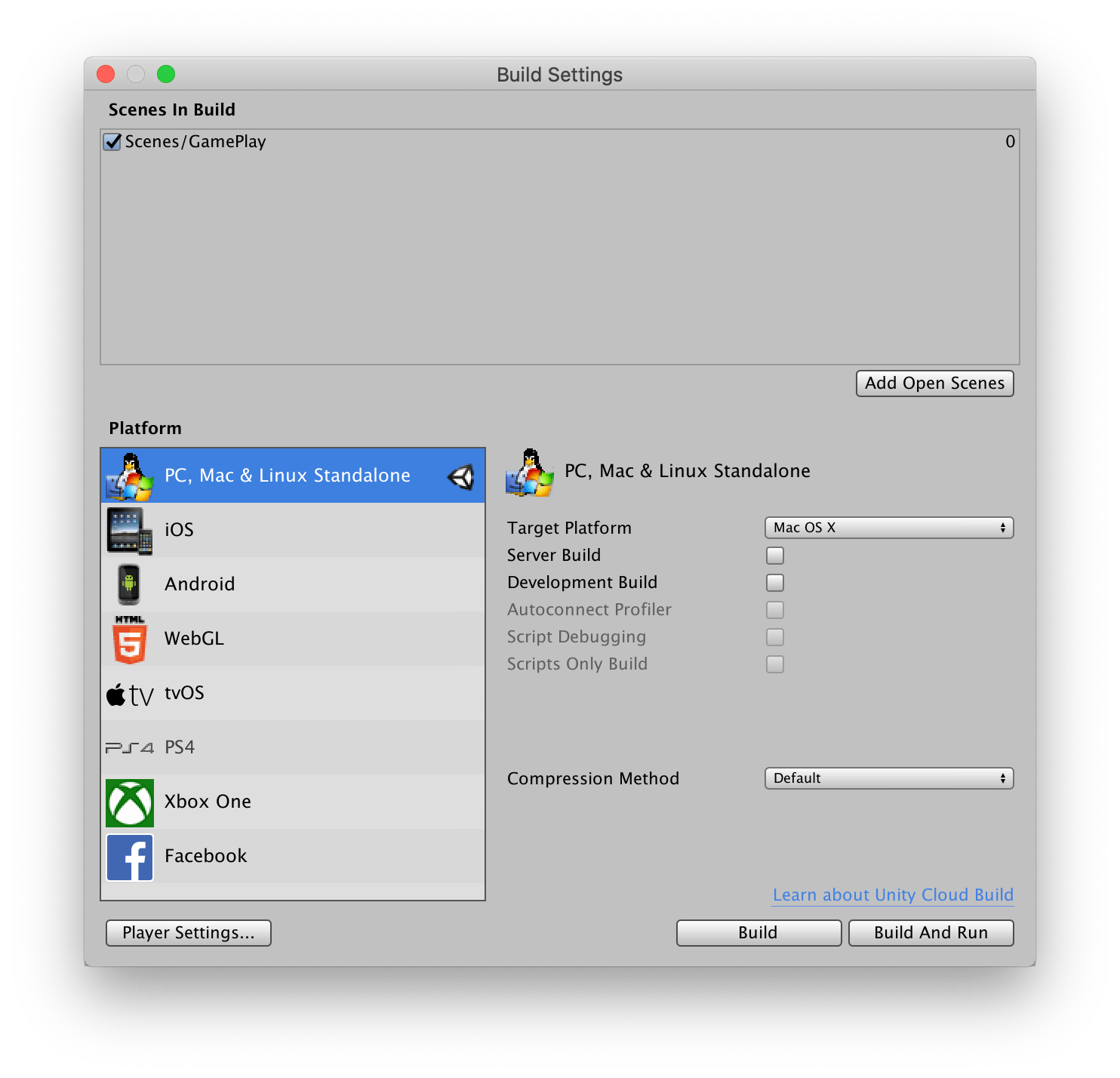
- Player Settings
下载
游戏
Release: https://github.com/GameDevLog/GameDevLogTemplete/releases