游戏展示
游戏开发
创建 2D 游戏
略
Main Camera
- Inspector
- Camera
- Background:
red
- Background:
- Camera
New Sprite
- Hierarchy
- Create
- Sprite:
Home
- Sprite:
- Create
- Inspector
- Transform: reset
- Scale
- Y:
3.8
- X:
3.8
- Y:
- Scale
- Sprite Renderer
- Sprite:
Knob
- Color:
black
alpha: 40 #00000028
- Sprite:
- Transform: reset
Player
- Hierarchy
- Create
- Sprite:
Player
- Sprite:
- Create
- Inspector
- Transform: reset
- Position
- Y:
0.6
- Y:
- Position
- Sprite Renderer
- Sprite:
Knob
- Sprite:
- Rigidbody 2D
- Body Type
- Kinematic
- Body Type
- Circle Collider 2D
- Is Trigger:
True
- Is Trigger:
- Transform: reset
Script
Player.cs
1 | using UnityEngine; |
2 | |
3 | public class Player : MonoBehaviour |
4 | { |
5 | public float moveSpeed = 600f; |
6 | private float movement; |
7 | |
8 | void Update() |
9 | { |
10 | movement = Input.GetAxis("Horizontal"); |
11 | } |
12 | |
13 | private void FixedUpdate() |
14 | { |
15 | transform.RotateAround(Vector3.zero, Vector3.back, movement * Time.fixedDeltaTime * moveSpeed); |
16 | } |
17 | } |
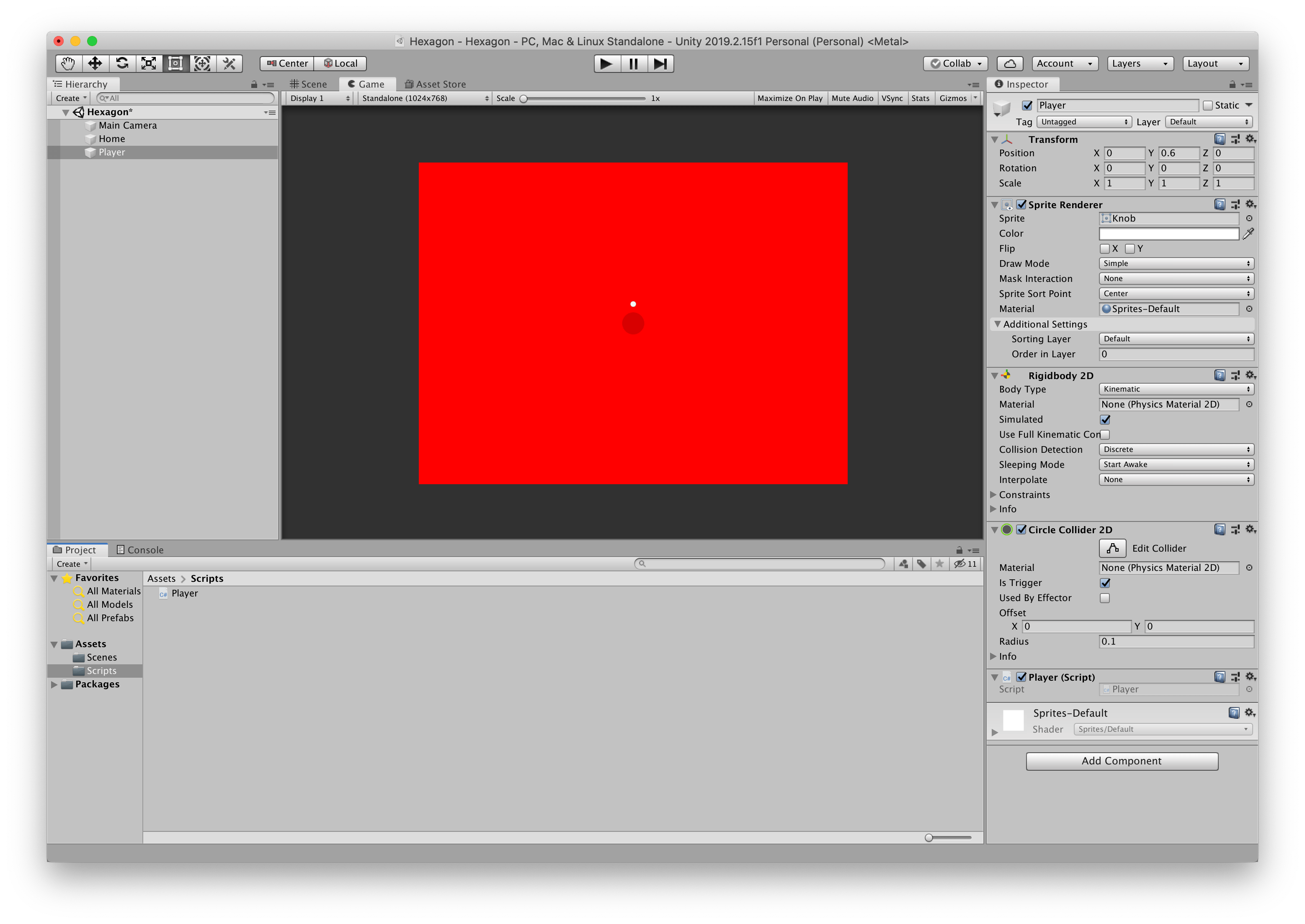
Hexagon
- Hierarchy
- Create
- Effects
- Line:
Hexagon
- Line:
- Effects
- Create
- Inspector
- Position
- Size:
6
- Size:
- Position
Index | X | Y | Z |
---|---|---|---|
0 | -0.5 | 0.8 | 0 |
1 | -0.95 | 0 | 0 |
2 | -0.5 | -0.8 | 0 |
3 | 0.5 | -0.8 | 0 |
4 | 0.95 | 0 | 0 |
5 | 0.5 | 0.8 | 0 |
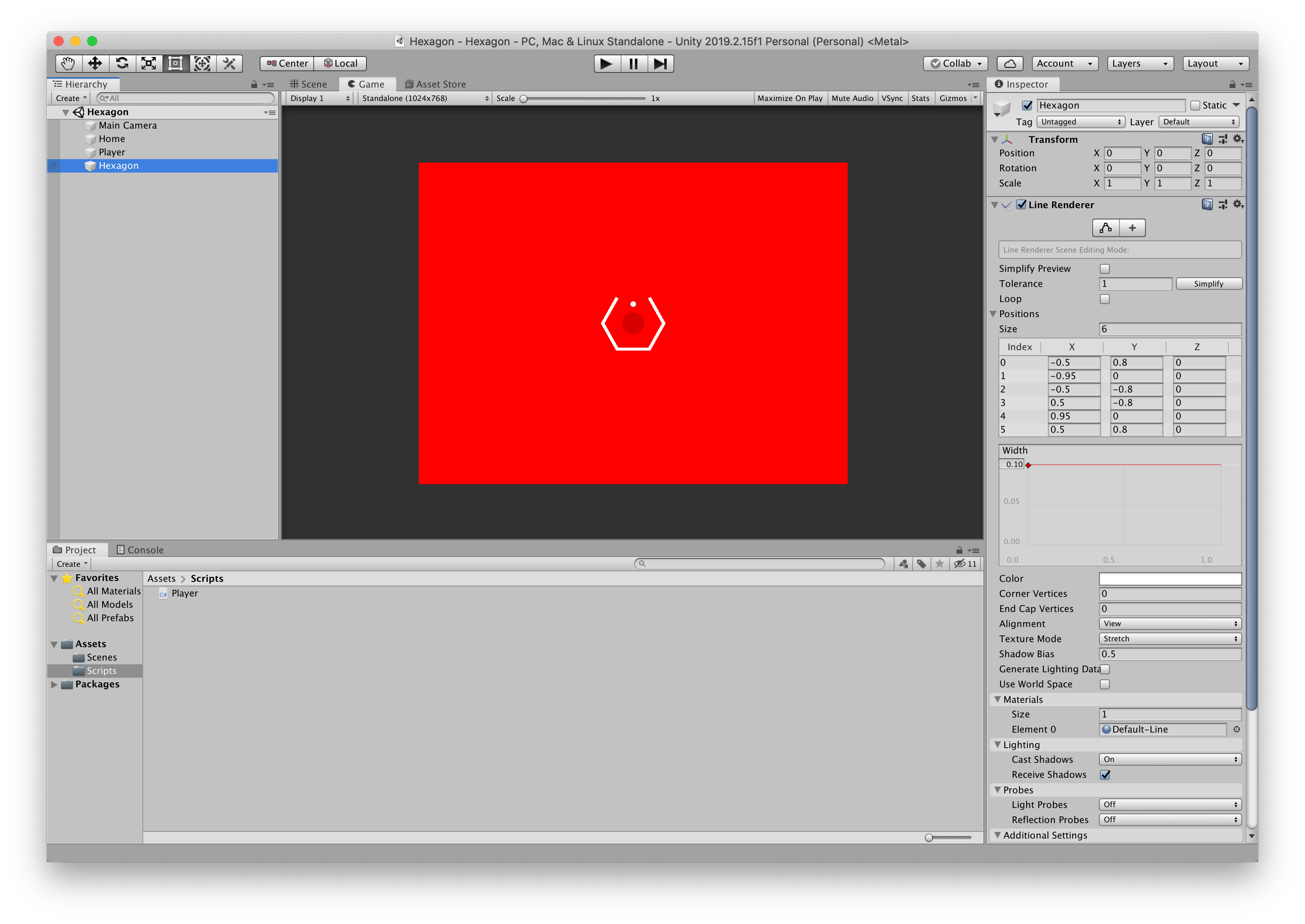
Collider
- Hierarchy
- Hexagon
- Create
- Empty:
Collider
- Empty:
- Create
- Hexagon
- Edge Collider 2D
- Position
- Size:
6
- Size:
- Position
Index | X | Y |
---|---|---|
0 | -0.5 | 0.8 |
1 | -0.95 | 0 |
2 | -0.5 | -0.8 |
3 | 0.5 | -0.8 |
4 | 0.95 | 0 |
5 | 0.5 | 0.8 |
Hexagon.cs
1 | using UnityEngine; |
2 | |
3 | public class Hexagon : MonoBehaviour |
4 | { |
5 | public Rigidbody2D rb; |
6 | public float shrinkSpeed = 3f; |
7 | |
8 | void Start() |
9 | { |
10 | rb.rotation = Random.Range(0f, 360f); |
11 | transform.localScale = Vector3.one * 10f; |
12 | } |
13 | |
14 | void Update() |
15 | { |
16 | transform.localScale -= Vector3.one * shrinkSpeed * Time.deltaTime; |
17 | |
18 | if (transform.localScale.x < 0.05f) |
19 | { |
20 | Destroy(gameObject); |
21 | } |
22 | } |
23 | } |
Frefabs / 预置体
将 Hexagon
作成 Frefabs
Spawner / 生成器
- Hierarchy
- Create
- Empty:
Spawner
- Empty:
- Create
1 | using UnityEngine; |
2 | |
3 | public class Spawner : MonoBehaviour |
4 | { |
5 | public float spawnRate = 1f; |
6 | public GameObject hexoagonPrefab; |
7 | private float nextTimeToSpawn; |
8 | |
9 | void Update() |
10 | { |
11 | if (Time.time >= nextTimeToSpawn) |
12 | { |
13 | Instantiate(hexoagonPrefab, Vector3.zero, Quaternion.identity); |
14 | nextTimeToSpawn = Time.time + 1f / spawnRate; |
15 | } |
16 | } |
17 | } |
Restart / 重玩
1 | diff --git a/Assets/Scripts/Player.cs b/Assets/Scripts/Player.cs |
2 | index 5887c5c..723bbc4 100644 |
3 | --- a/Assets/Scripts/Player.cs |
4 | +++ b/Assets/Scripts/Player.cs |
5 |
|
6 | <U+FEFF>using UnityEngine; |
7 | +using UnityEngine.SceneManagement; |
8 | |
9 | public class Player : MonoBehaviour |
10 | { |
11 | @@ -14,4 +15,9 @@ public class Player : MonoBehaviour |
12 | { |
13 | transform.RotateAround(Vector3.zero, Vector3.back, movement * Time.fixedDeltaTime * moveSpeed); |
14 | } |
15 | + |
16 | + private void OnTriggerEnter2D(Collider2D collision) |
17 | + { |
18 | + SceneManager.LoadScene(SceneManager.GetActiveScene().buildIndex); |
19 | + } |
20 | } |
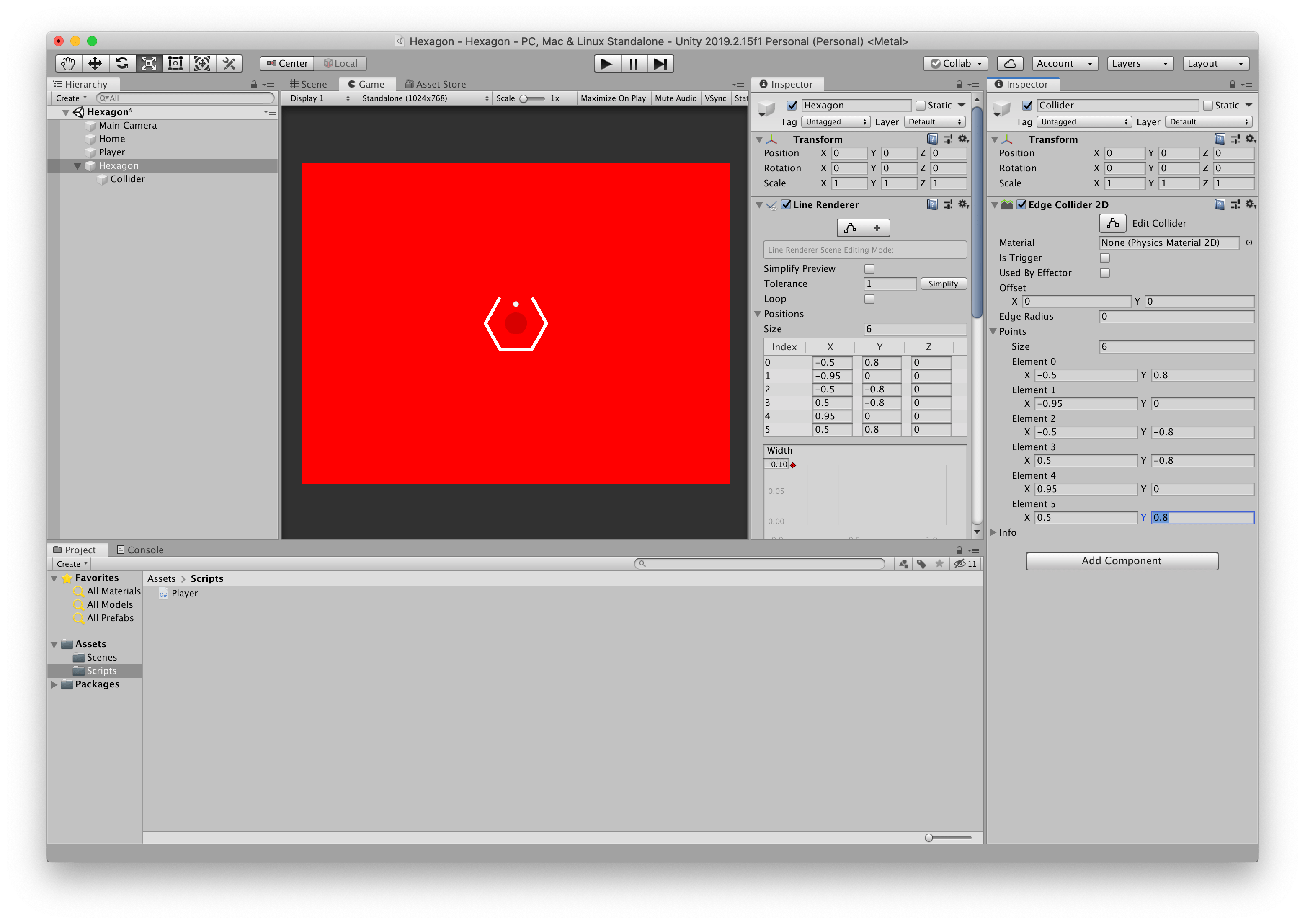
Camera rotate
1 | using System.Collections; |
2 | using UnityEngine; |
3 | |
4 | public class Rotator : MonoBehaviour |
5 | { |
6 | void Update() |
7 | { |
8 | transform.Rotate(Vector3.forward, Time.deltaTime * 30f); |
9 | } |
10 | } |
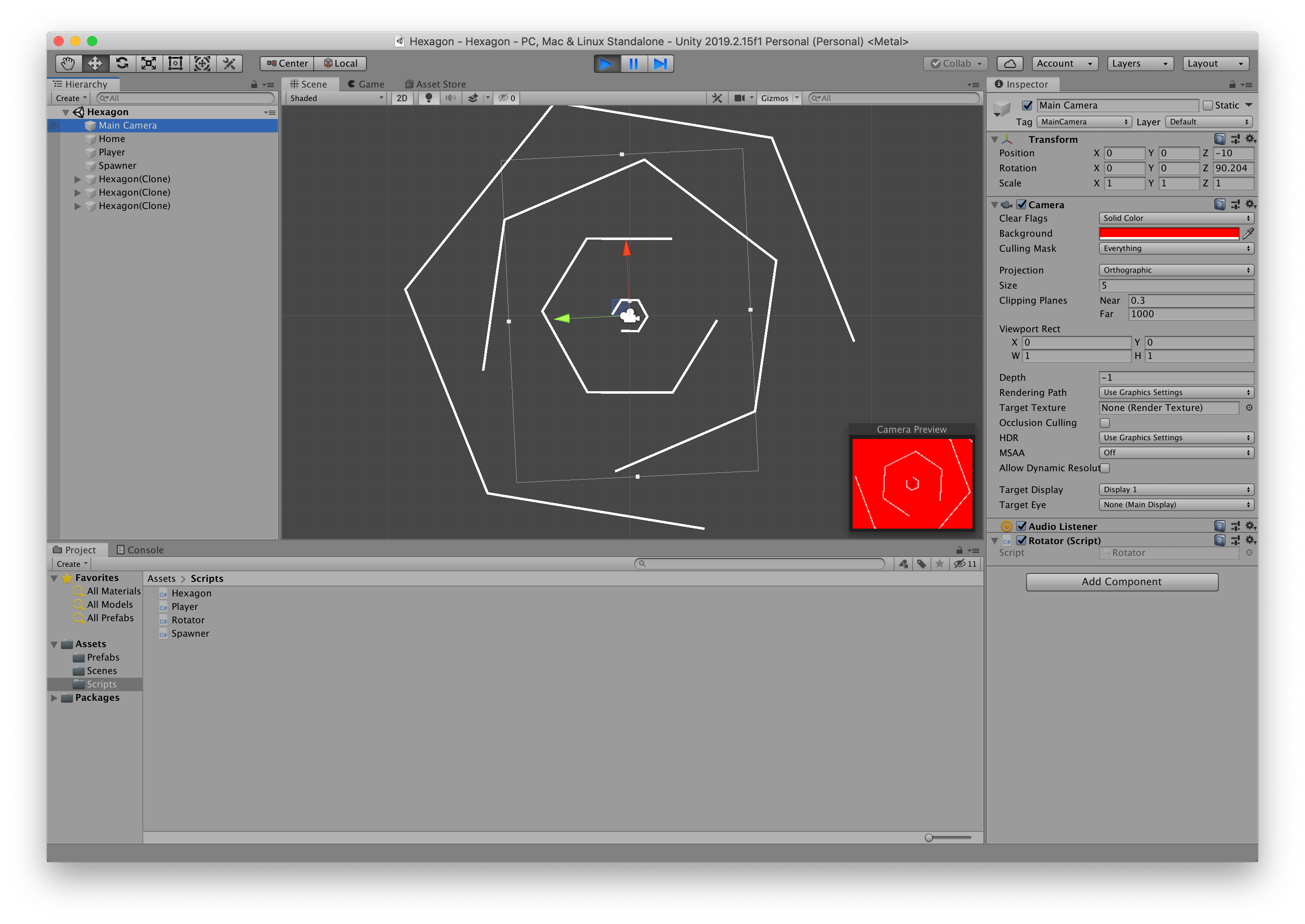
小结
- Dynamic
- Static 不移动
- kinematic 运动的, 不受重力影响
下载
游戏
Release: https://github.com/GameDevLog/GameDevLogTemplete/releases