游戏展示
游戏开发
创建 2D 游戏
略
Import Assets / 导入资源
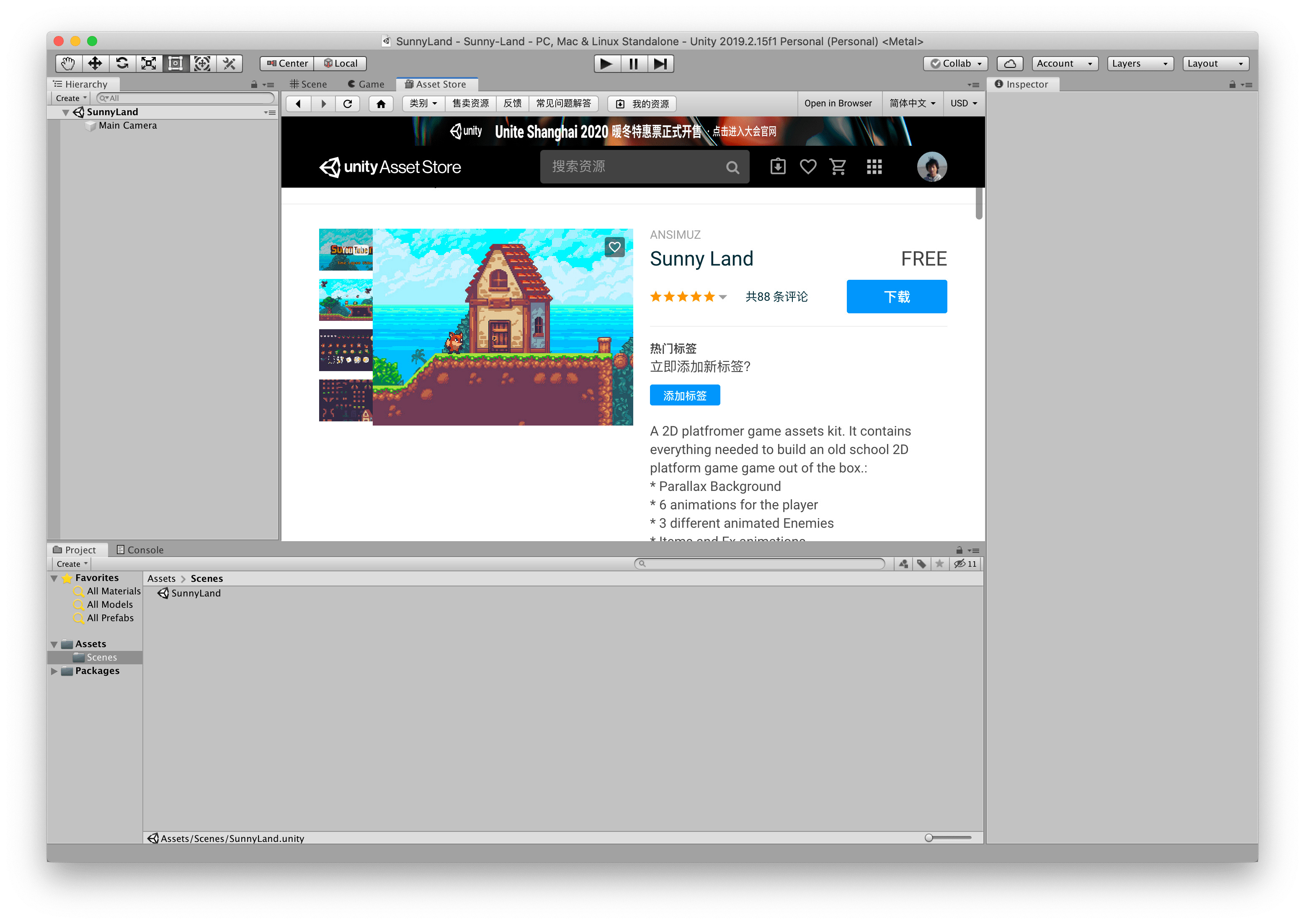
Background / 背景
每单元像素改为 16
,以下所有资源做相同操作。
Project
- Sunnyland
- artwork
- Environment
- back
- Environment
- artwork
- Sunnyland
Inspector
- Sprite Mode
- Pixels Per Unit:
16
- Pixels Per Unit:
- Sprite Mode
back -> Hierarchy
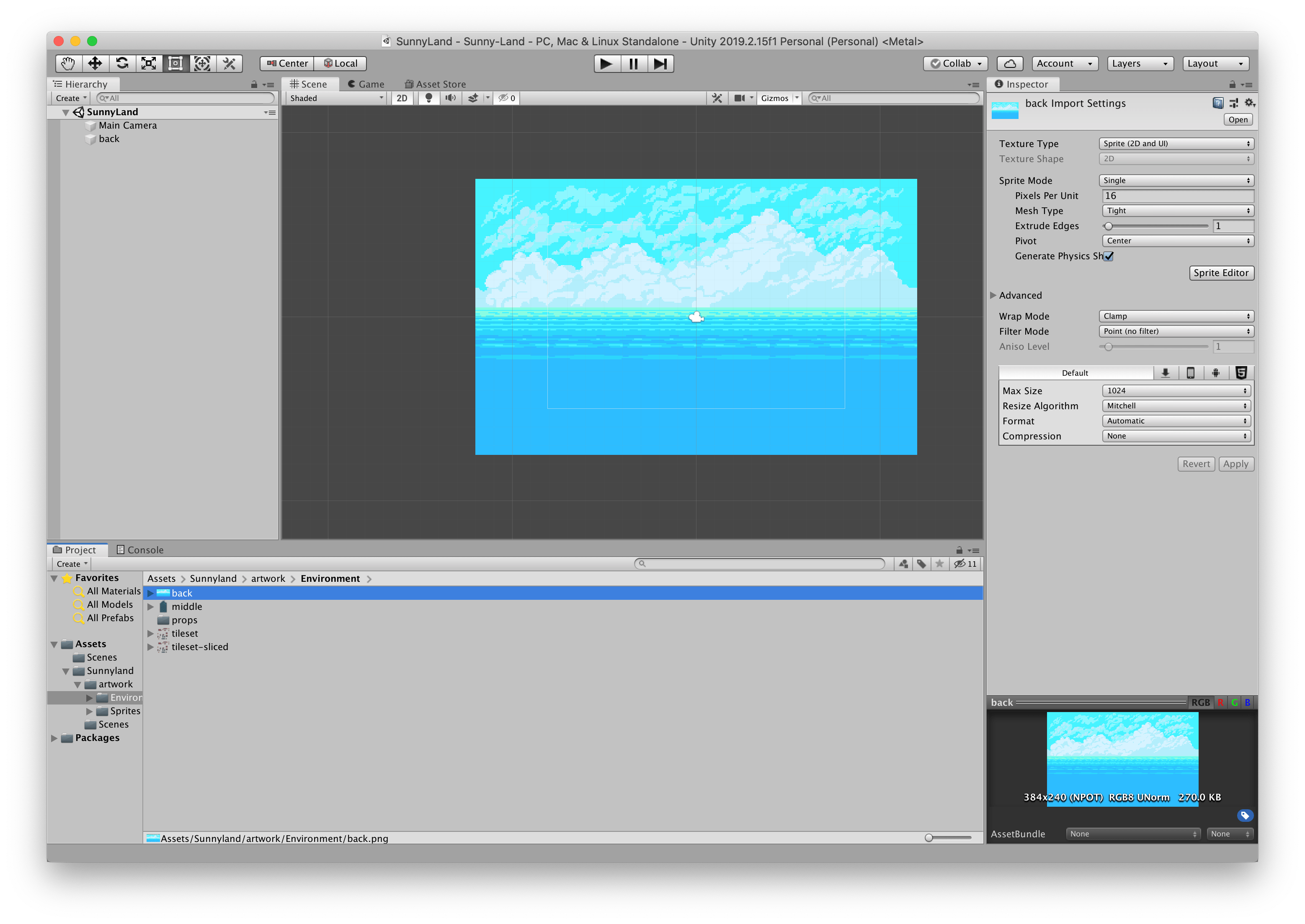
Tilemap / 瓦片地图
- Hierarchy
- Create
- 2D Object
- Tilemap
- 2D Object
- Create
- Window
- 2D
- Tile palette
- 2D
- Tile palette
- Create New Palette
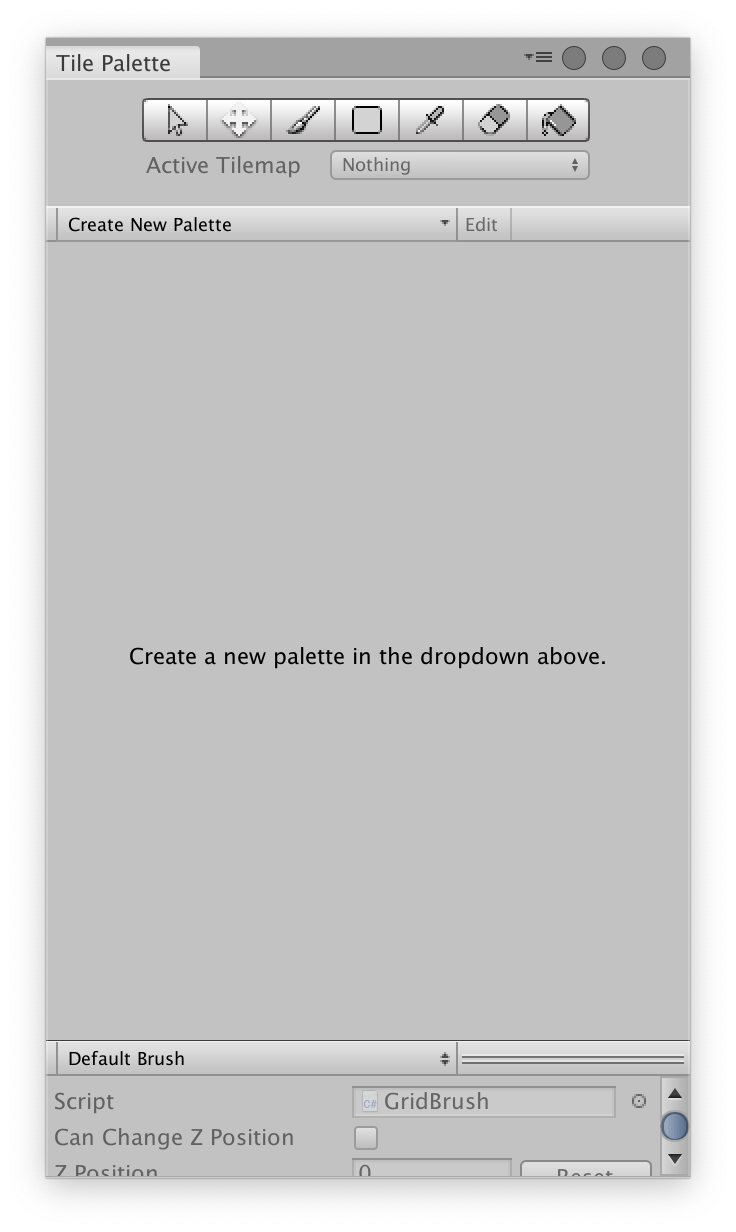
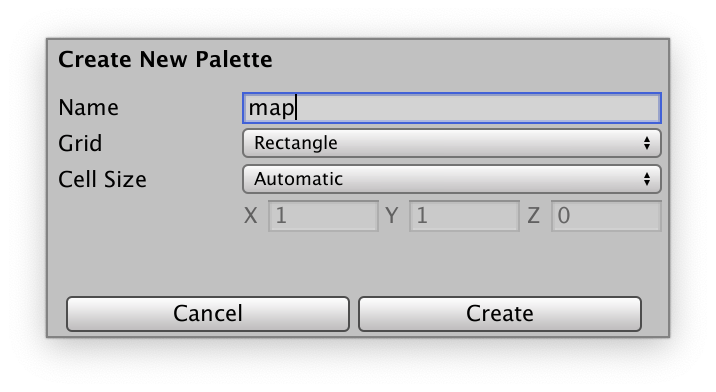
- Project
- Sunnyland
- artwork
- Environment
- tileset
- Environment
- artwork
- Sunnyland
- Inspector
- Sprite Mode:
Multiple
- Pixels Per Unit:
16
- Pixels Per Unit:
- Apply
- Sprite Editor
- Sprite Mode:
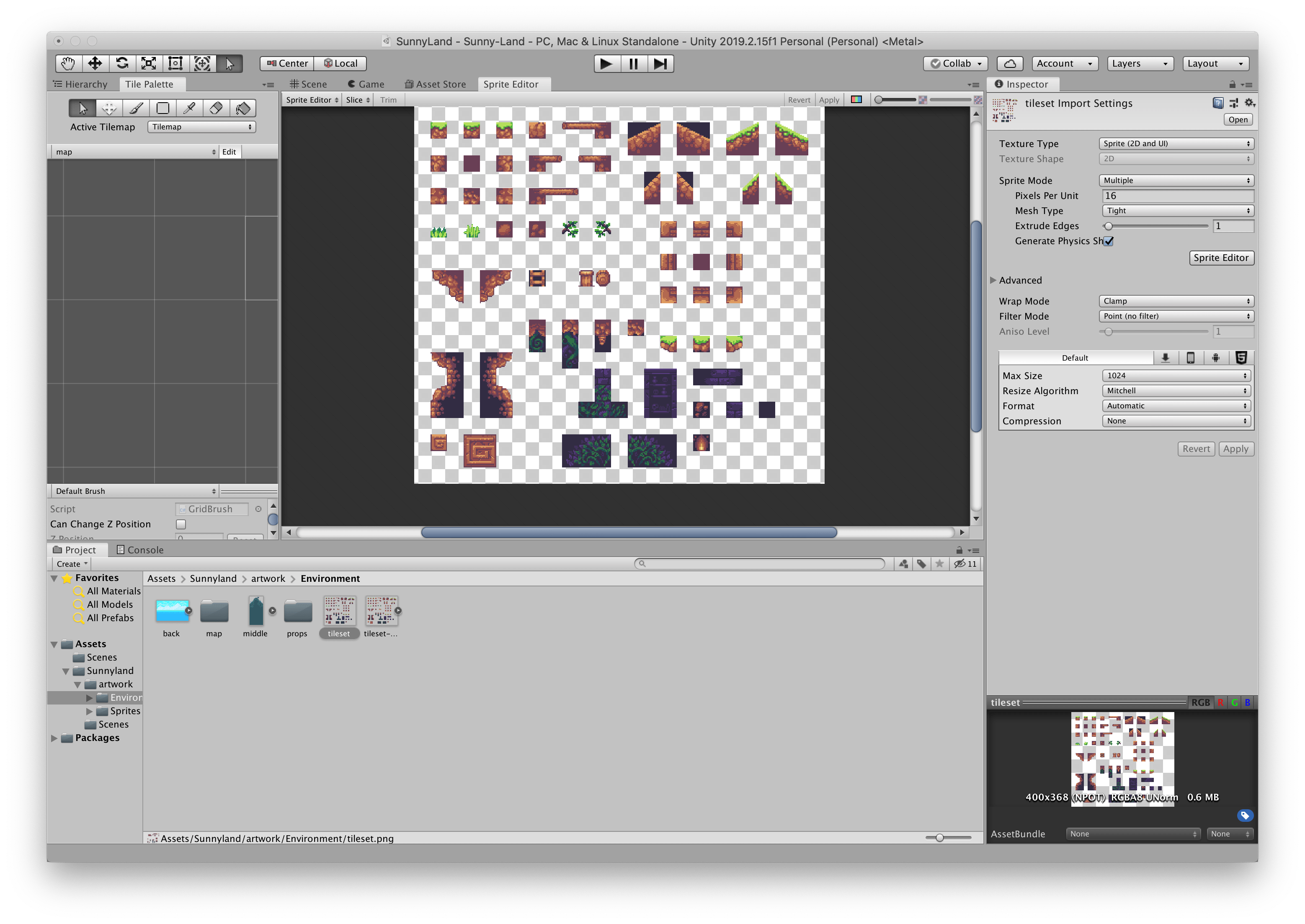
- Sprite Editor
- Slice
- Type:
Grid By Cell Size
- Pixel Size
- X:
16
- Y:
16
- X:
- Type:
- Apply
- Slice
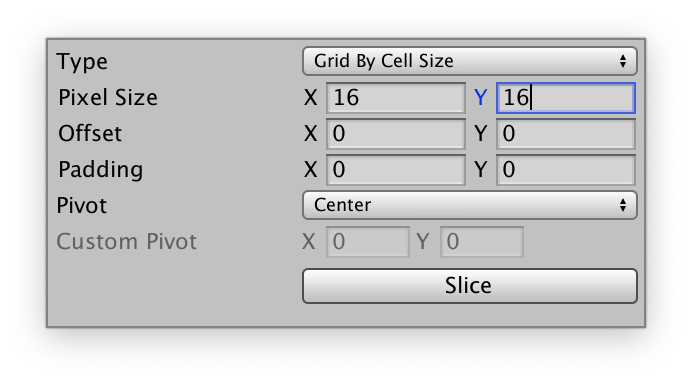
- Project
- Sunnyland
- artwork
- Environment
- tileset ->
Tile Palette
- tileset ->
- Environment
- artwork
- Sunnyland
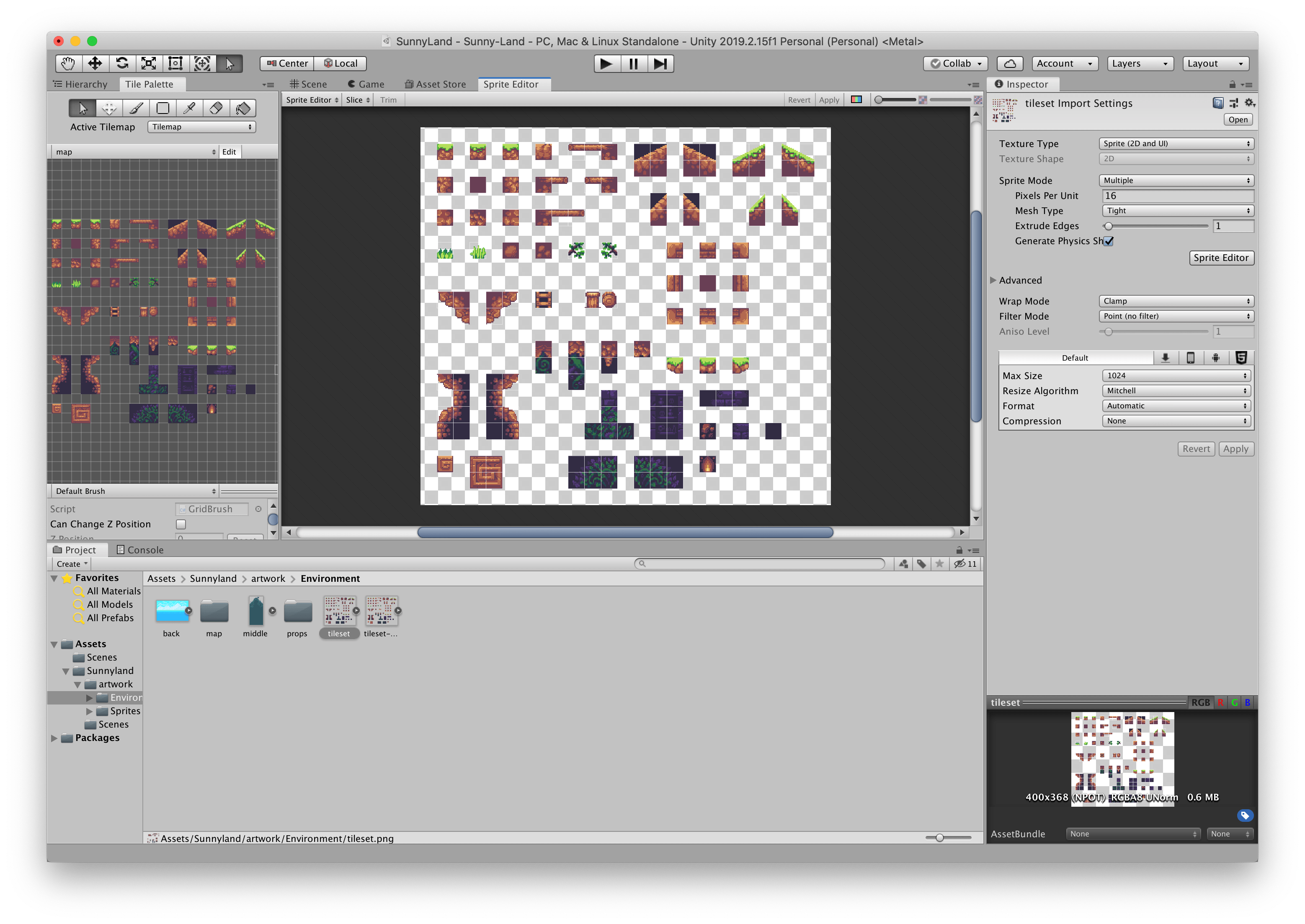
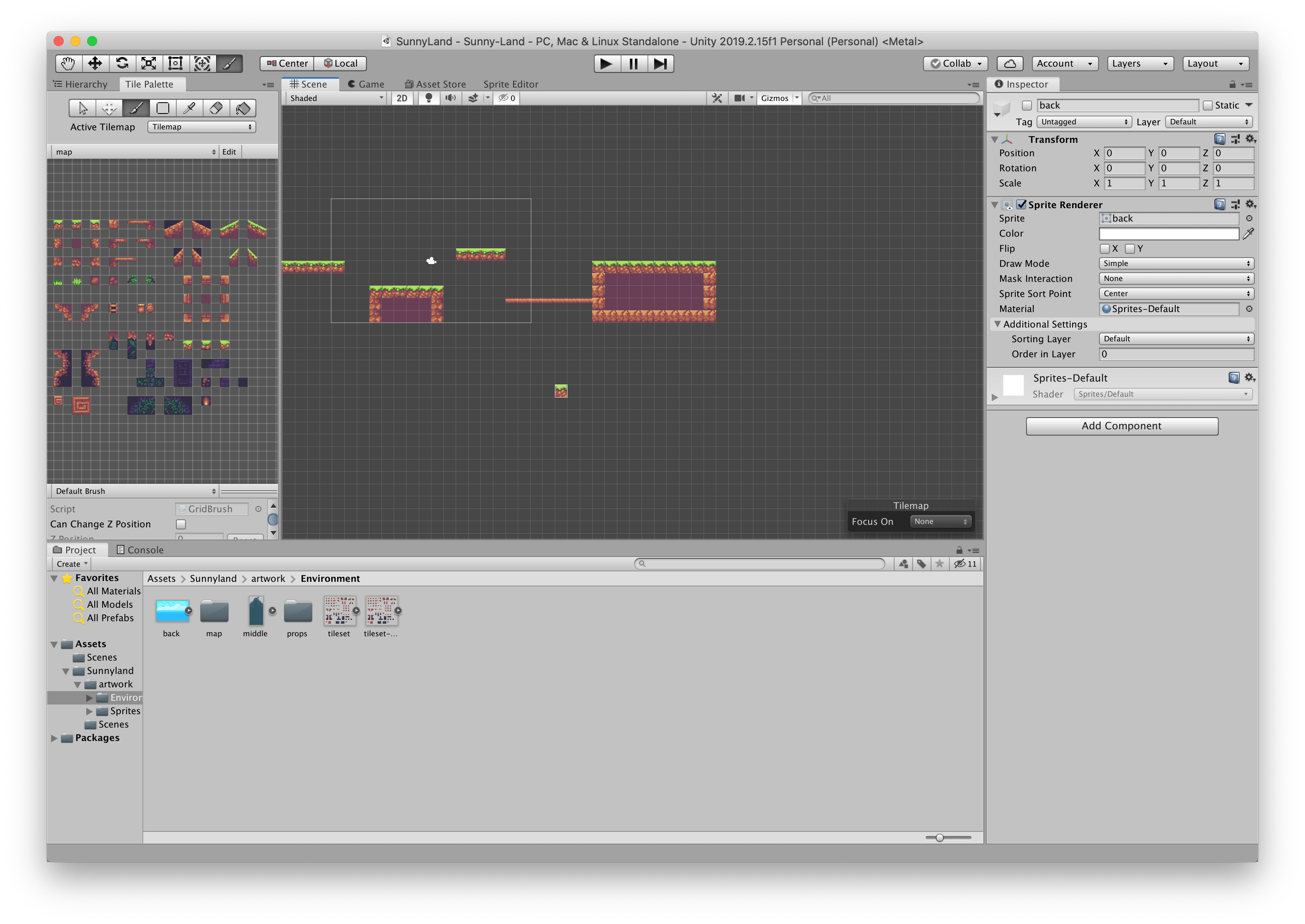
Main Camera Size / 调整相机尺寸
- Inspector
- Camera
- Size:
6
- Size:
- Camera
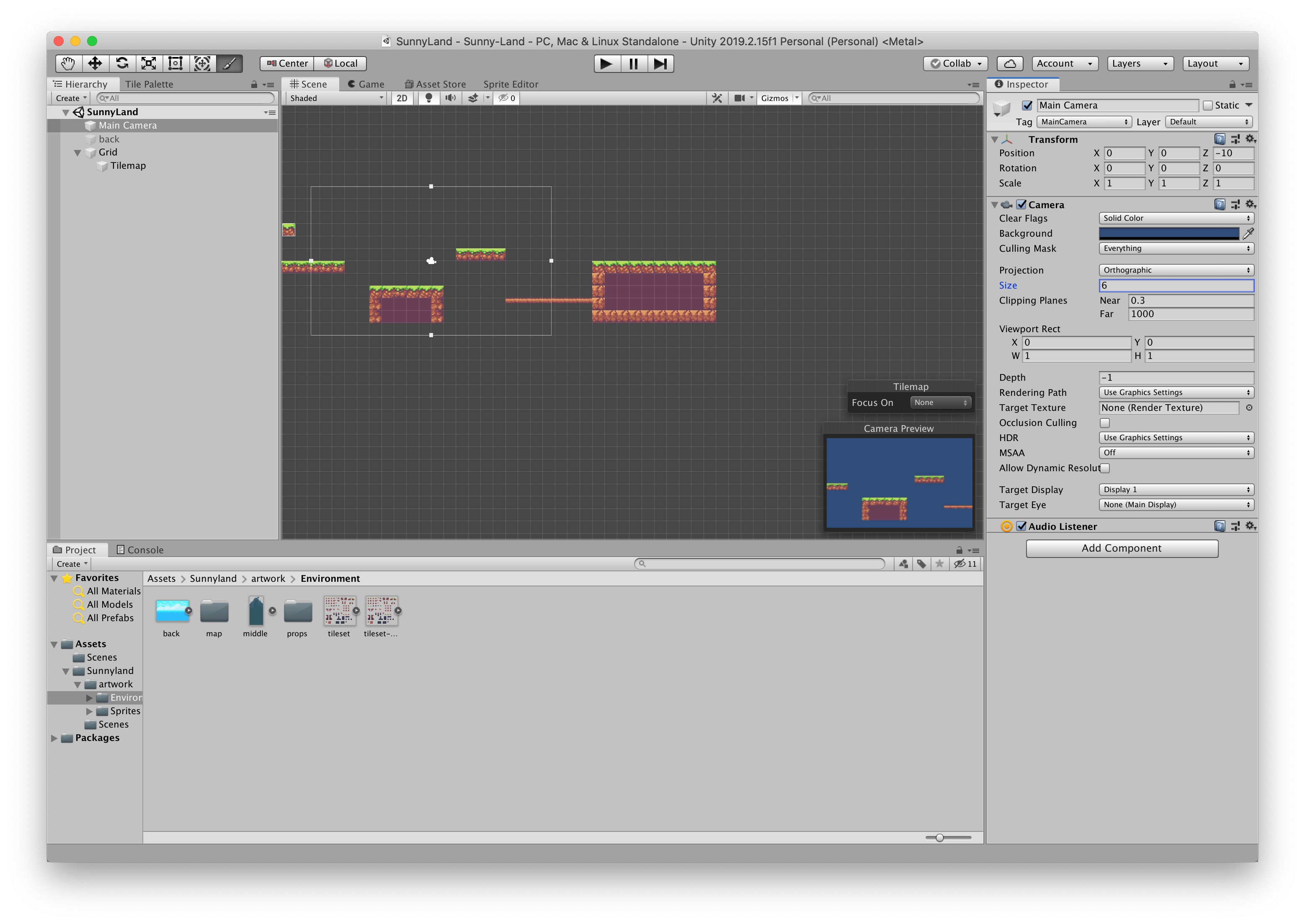
Sorting Layer / 层排序
Order In Layer
也可以排序,数字越大越前
- Hierarchy
- Back
- Inspector
- Sprite Renderer
- Additional Settings
- Sorting Layer
- Add Sorting Layer…
- Sorting Layer
- Additional Settings
- Sprite Renderer
新增
- Background
- Foreground
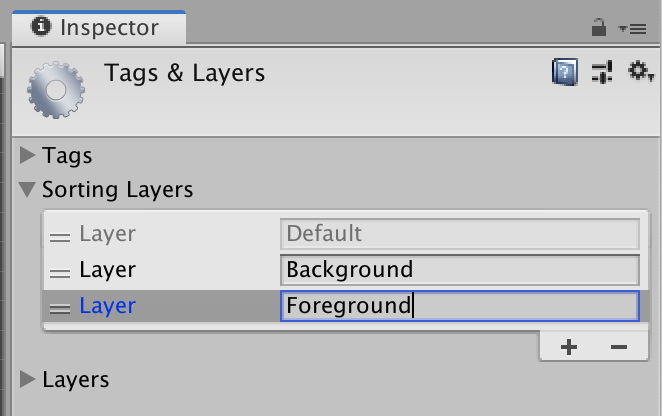
Hierarchy
- Back
Inspector
- Check:
显示 Back
- Sprite Renderer
- Additional Settings
- Sorting Layer:
Background
- Sorting Layer:
- Additional Settings
- Check:
Hierarchy
- Grid
- Tielmap
- Grid
Inspector
- Sprite Renderer
- Additional Settings
- Sorting Layer:
Fackground
- Sorting Layer:
- Additional Settings
- Sprite Renderer
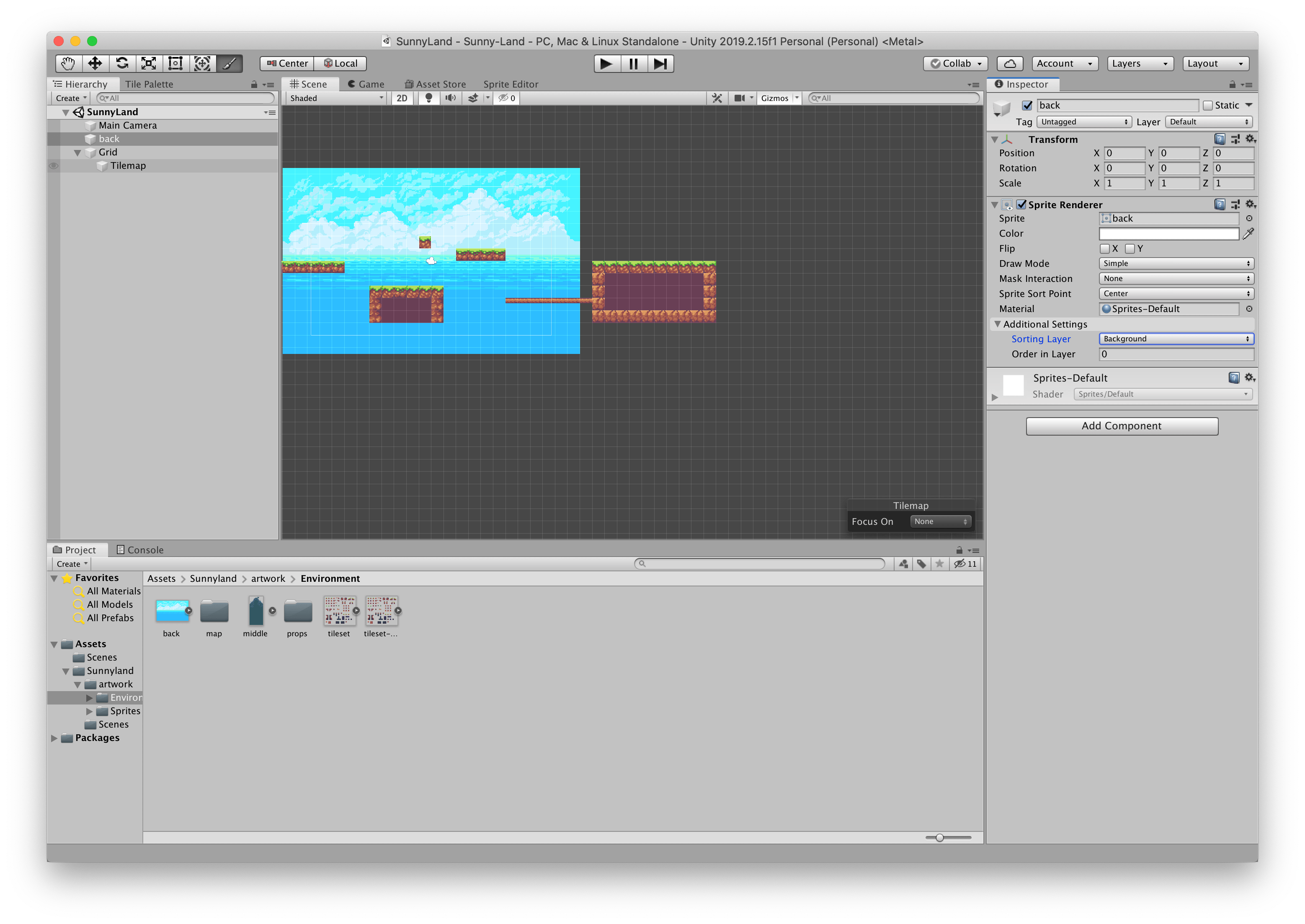
Player
创建 Player Sprite, 并将 player-idle-1
拖入
- Project
- Assets
- Sunnyland
- artwork
- Sprites
- player
- idle:
16 Pixels Per Unit
player-idle-1
- idle:
- player
- Sprites
- artwork
- Sunnyland
- Assets
->
- Hierarchy
- Create
- 2D Object
- Sprite:
Player
- Sprite:
- 2D Object
- Create
- Inspector
- Sprite Renderer
- Sprite:
player-idle-1
- Sprite:
- Sprite Renderer
Physics / 物理
Tilemap
- Inspector
- Tilemap Collider 2D
Player
- Inspector
- Rigidbody 2D
- Constraints
- Freeze Rotation
- Z:
true
# 不让 Z 轴移动
- Z:
- Freeze Rotation
- Constraints
- Box Collider 2D
- Edit Collider
- Rigidbody 2D
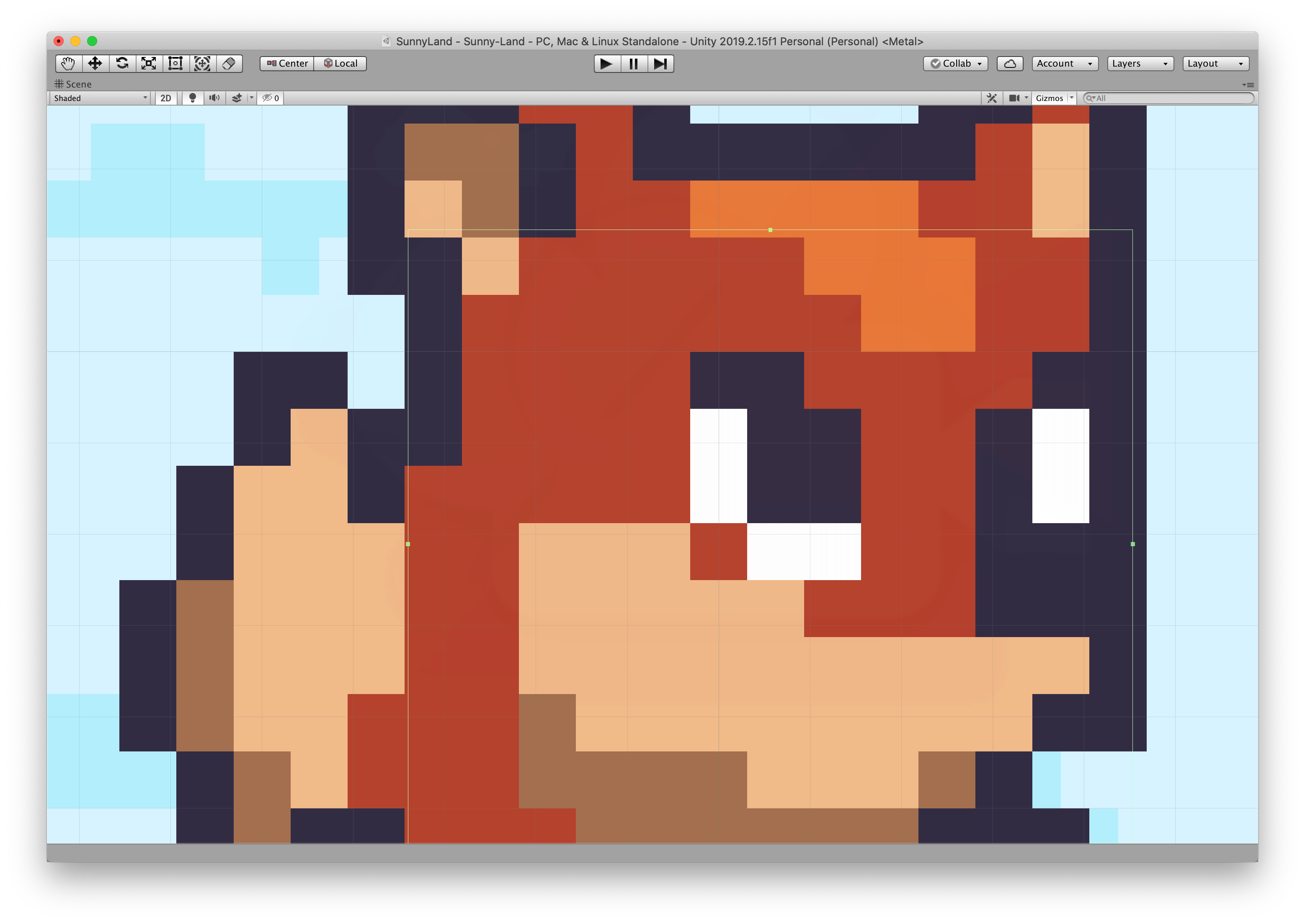
PlayerController.cs
1 | using System; |
2 | using UnityEngine; |
3 | |
4 | public class PlayerController : MonoBehaviour |
5 | { |
6 | public float moveSpeed; |
7 | public float jumpForce; |
8 | private Rigidbody2D rb; |
9 | |
10 | private void Start() |
11 | { |
12 | rb = GetComponent<Rigidbody2D>(); |
13 | |
14 | if (moveSpeed <= 0) |
15 | { |
16 | moveSpeed = 400; |
17 | } |
18 | |
19 | if (jumpForce <= 0) |
20 | { |
21 | jumpForce = 400; |
22 | } |
23 | } |
24 | |
25 | private void FixedUpdate() |
26 | { |
27 | Movement(); |
28 | } |
29 | |
30 | private void Movement() |
31 | { |
32 | // -1 ~ 1 |
33 | float horizontalMove = Input.GetAxis("Horizontal"); |
34 | // [-1, 0, 1] |
35 | float faceDirection = Input.GetAxisRaw("Horizontal"); |
36 | float EPSILON = 0.1f; |
37 | |
38 | if (Math.Abs(horizontalMove) > EPSILON) |
39 | { |
40 | rb.velocity = new Vector2(horizontalMove * moveSpeed * Time.deltaTime, rb.velocity.y); |
41 | } |
42 | |
43 | if ((Math.Abs(faceDirection) > EPSILON)) |
44 | { |
45 | transform.localScale = new Vector3(faceDirection, 1, 1); |
46 | } |
47 | |
48 | if (Input.GetButtonDown("Jump")) |
49 | { |
50 | rb.velocity = new Vector2(rb.velocity.x, jumpForce * Time.deltaTime); |
51 | } |
52 | } |
53 | } |
Animation / 动画
先选中 Player
先选中 Player
先选中 Player
- Porject
- Assets
- Animation
- Player
- Animator Controller:
Player
- Animator Controller:
- Player
- Animation
- Assets
->
- Inpsector
- Animator
- Controller:
Player
- Controller:
- Animator
- Hierarchy
- Player
- Window
- Animation
- Animation
- Animation
- Animation
- Create:
Idle
- Setting
- Show Sample Rate:
true
- Show Sample Rate:
- Samples:
10
- Create:
- Project
- Assets
- Sunnyland
- artwork
- Sprites
- player
- idle
- player-idle-[1-4] ->
Idle
- player-idle-[1-4] ->
- idle
- player
- Sprites
- artwork
- Sunnyland
- Assets
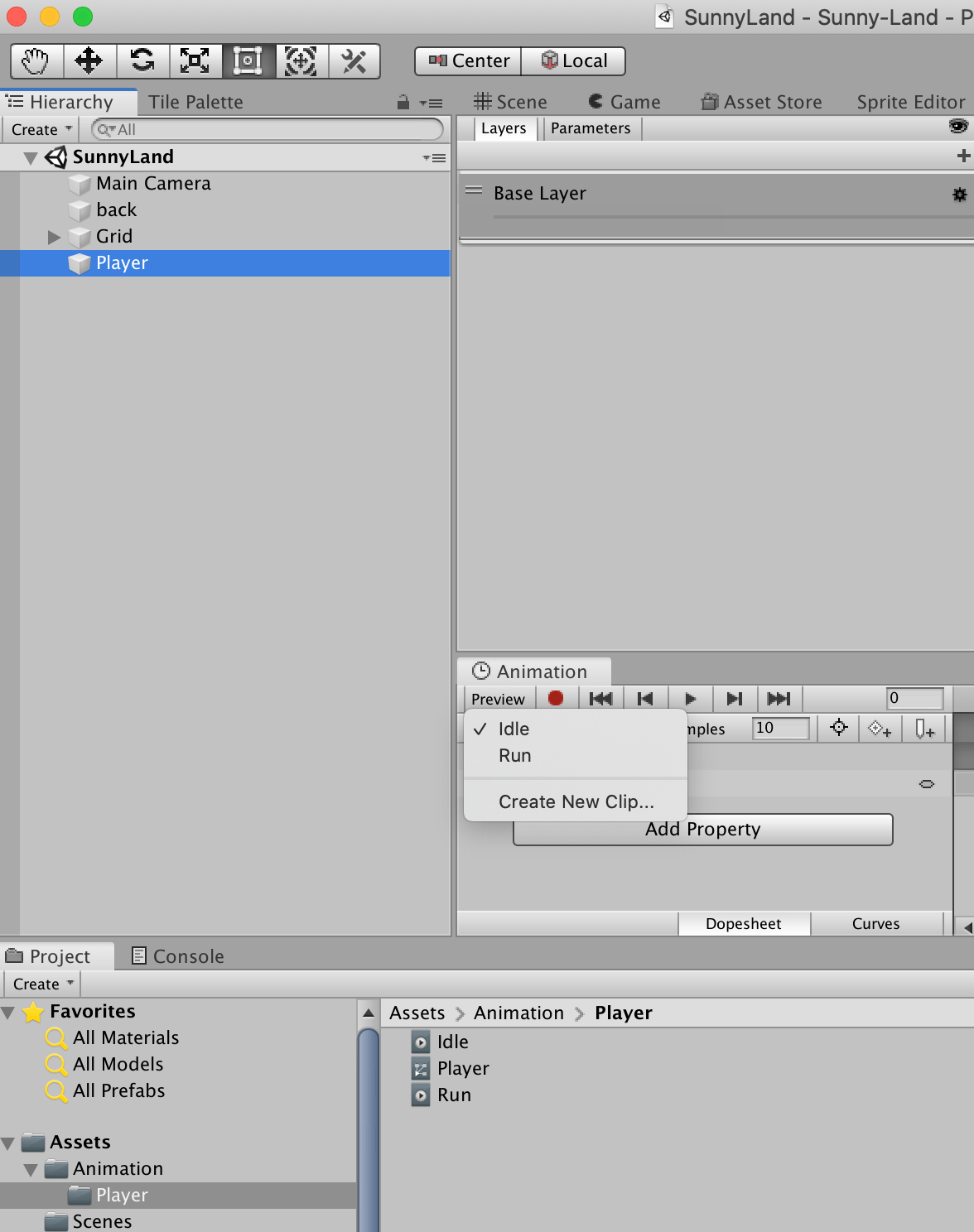
Animator / 动画师(动画转变)
- Window
- Animation
- Animator
- Animation
- Animator
- Idle
- Make Transition
- Run
- Jump
- Make Transition
- Run
- Make Transition
- Idle
- Jump
- Make Transition
- Jump
- Make Transition
- Fall
- Make Transition
- Fall
- Make Transition
- Idle
- Make Transition
- Parameters + float
- running:
0.0
- running:
- Parameters + bool
- jumping:
false
- falling:
false
- idle:
false
- jumping:
- Idle
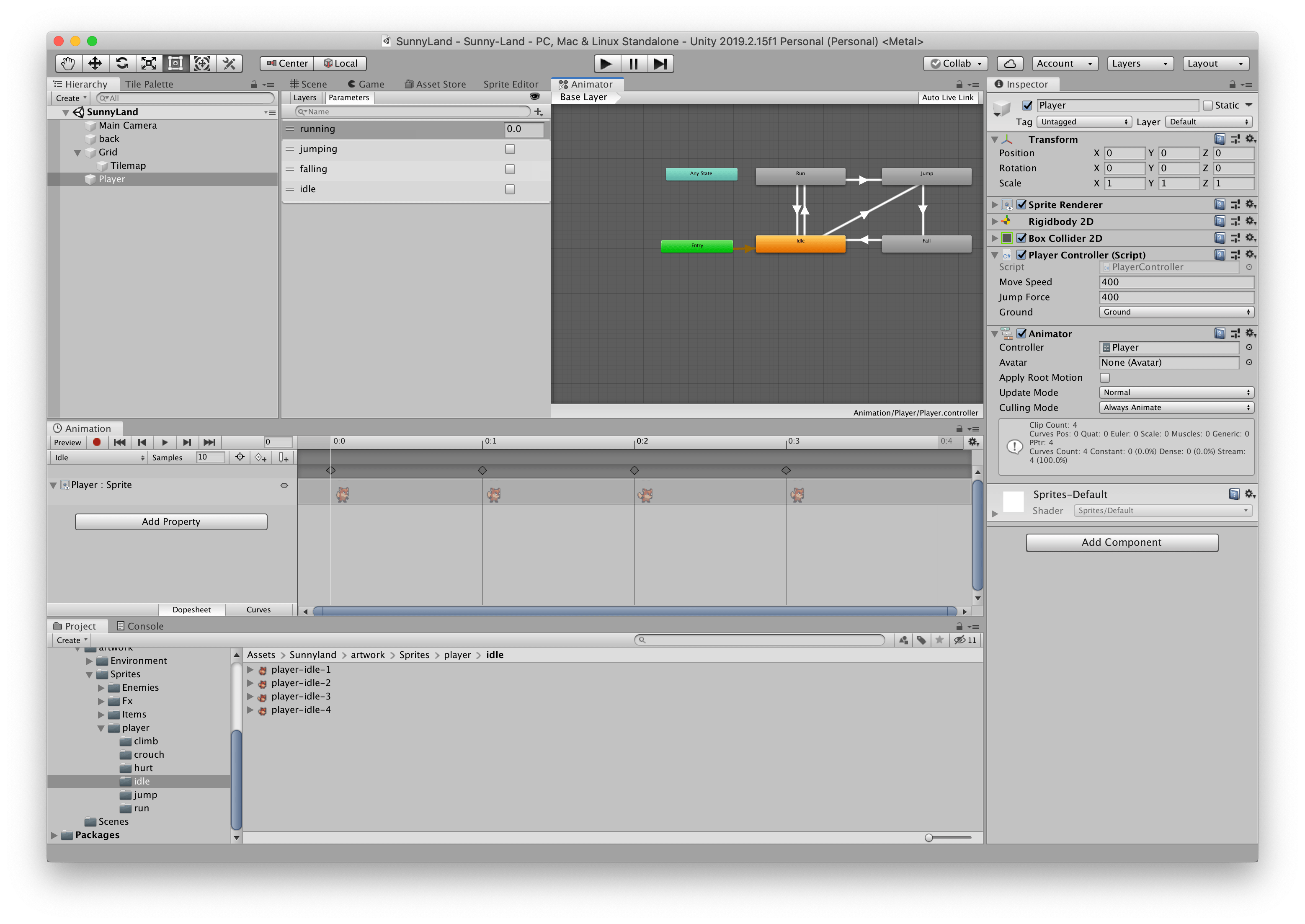
Idle -> Run
- Inspector
- Has Exit Time:
false
- Settings
- Transition Duration:
0
- Transition Duration:
- Conditions
- running >
0.1
- running >
- Has Exit Time:
Run -> Idle
- Inspector
- Has Exit Time:
false
- Settings
- Transition Duration:
0
- Transition Duration:
- Conditions
- running <
0.1
- running <
- Has Exit Time:
Idle -> Jump & Run -> Jump
- Inspector
- Has Exit Time:
false
- Settings
- Transition Duration:
0
- Transition Duration:
- Conditions
- jumping:
true
- jumping:
- Has Exit Time:
Jump -> Fall
- Inspector
- Has Exit Time:
false
- Settings
- Transition Duration:
0
- Transition Duration:
- Conditions
- falling:
true
- jumping:
false
- falling:
- Has Exit Time:
Fall -> Idle
- Inspector
- Has Exit Time:
false
- Settings
- Transition Duration:
0
- Transition Duration:
- Conditions
- falling:
false
- idle:
true
- falling:
- Has Exit Time:
Bug Fix:
- Bug 现象
Player
会卡住
- 原因
Box Collider 2D
与Tilemap
多点碰撞检测
- 解决方案
- 添加
Circle Collider 2D
,单点碰撞检测
- 添加
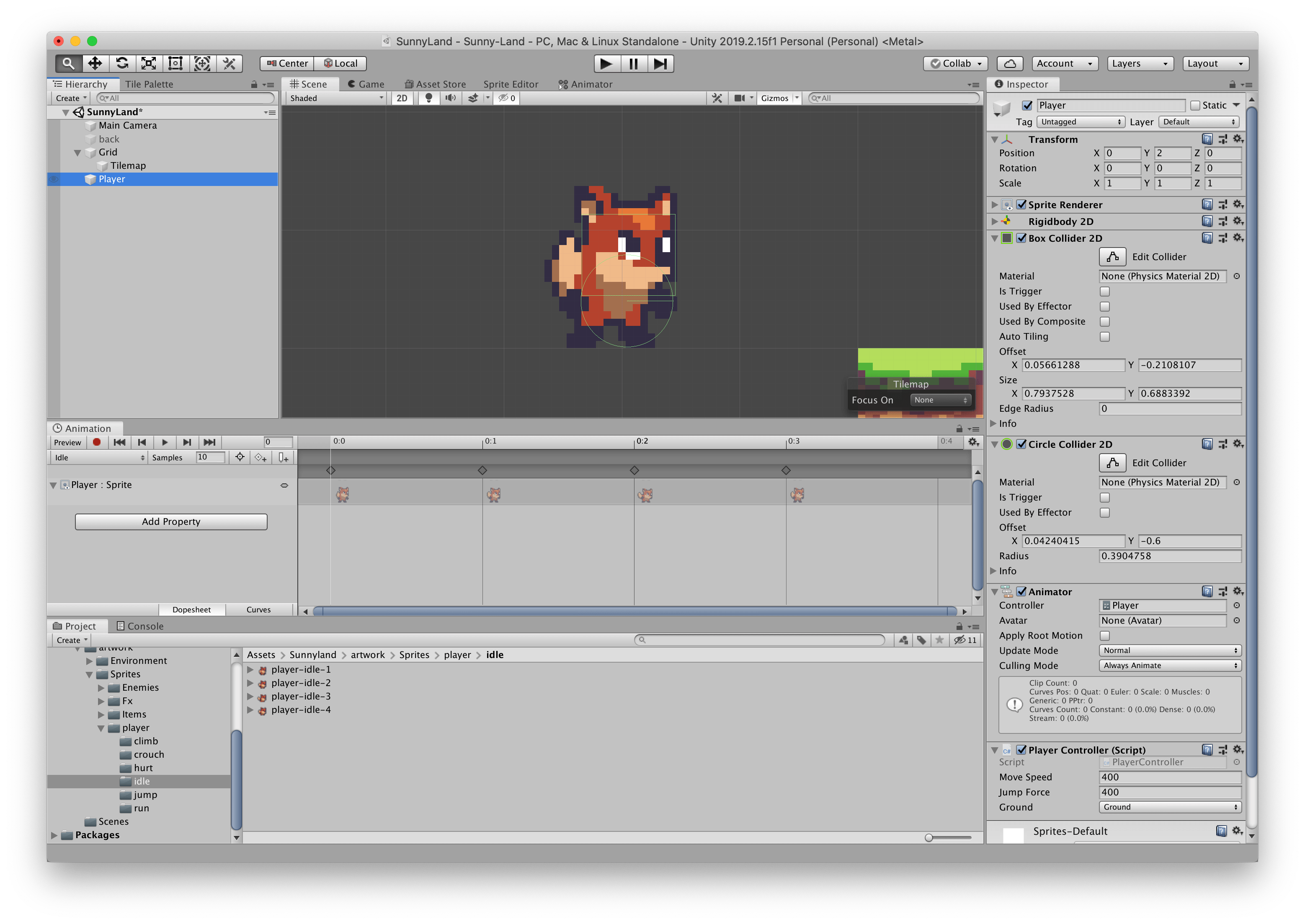
Cinemachine / 电影镜头
Install Package / 安装包
- Menu
- Window
- Package Manager
- Window
- Packages
- Cinemachine:
Install
- Cinemachine:
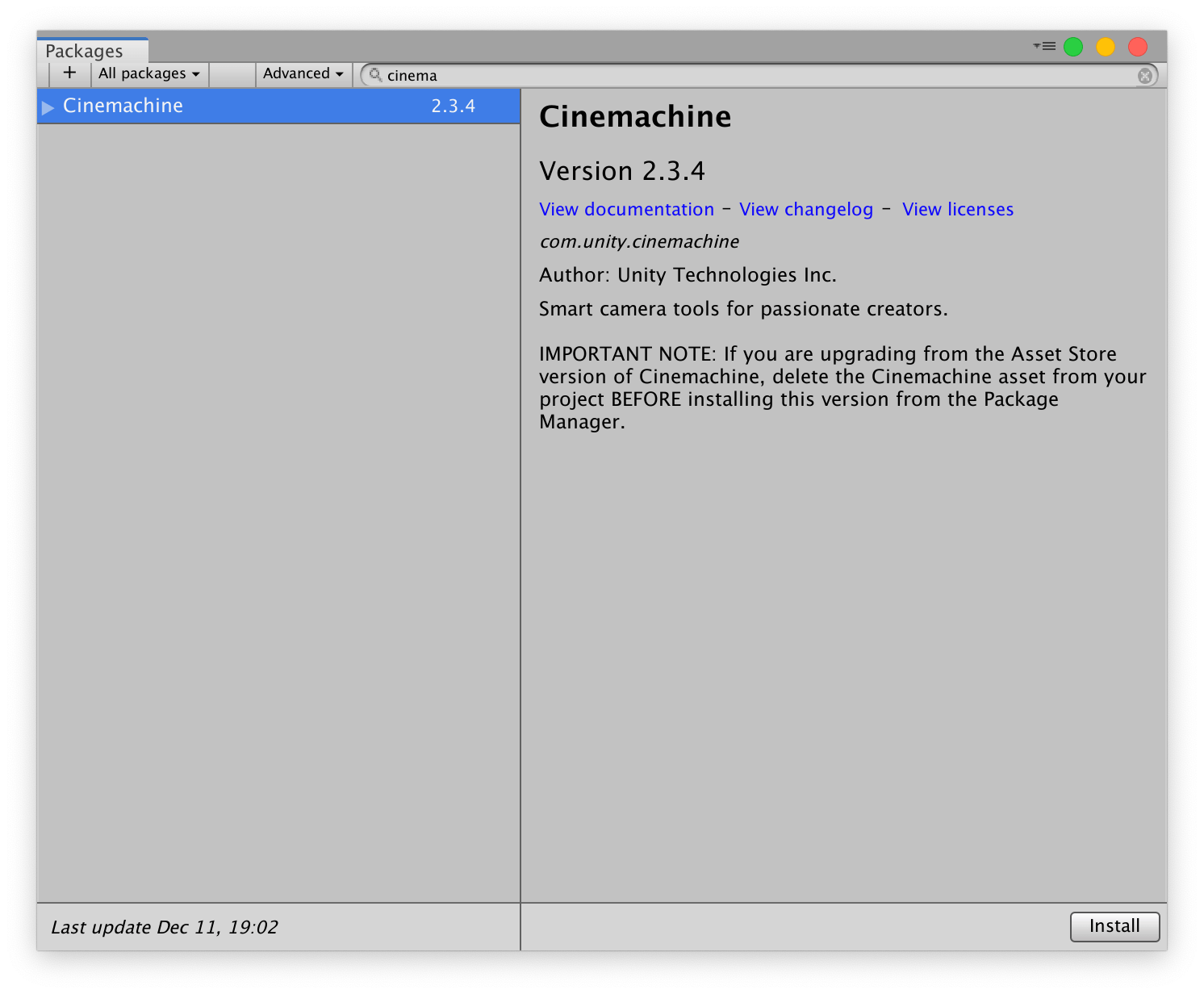
Create 2D Camera / 创建 2D Camera
- Menu
- Cinemachine
- Create 2D Camera
- Cinemachine
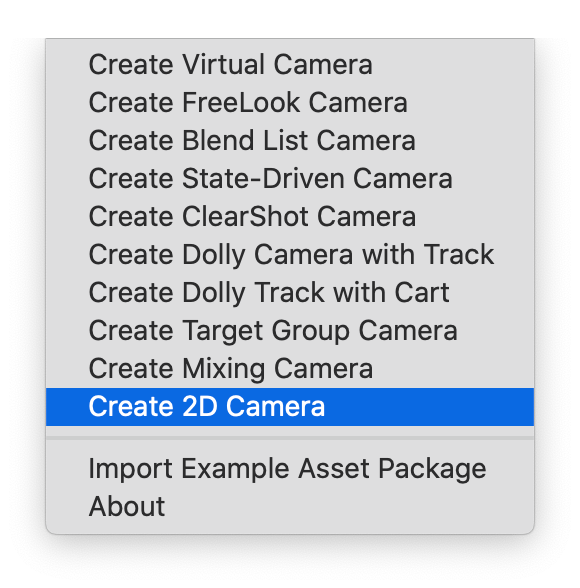
CM vcam1
- Inspector
- Cinemachine Virtual Camera(Script)
- Follow:
Player
- Lens
- Orthographic Size:
7.43
- Orthographic Size:
- Body
- Dead Zone Width:
0.2
- Dead Zone Height:
0.24
- Dead Zone Width:
- Follow:
- Cinemachine Virtual Camera(Script)
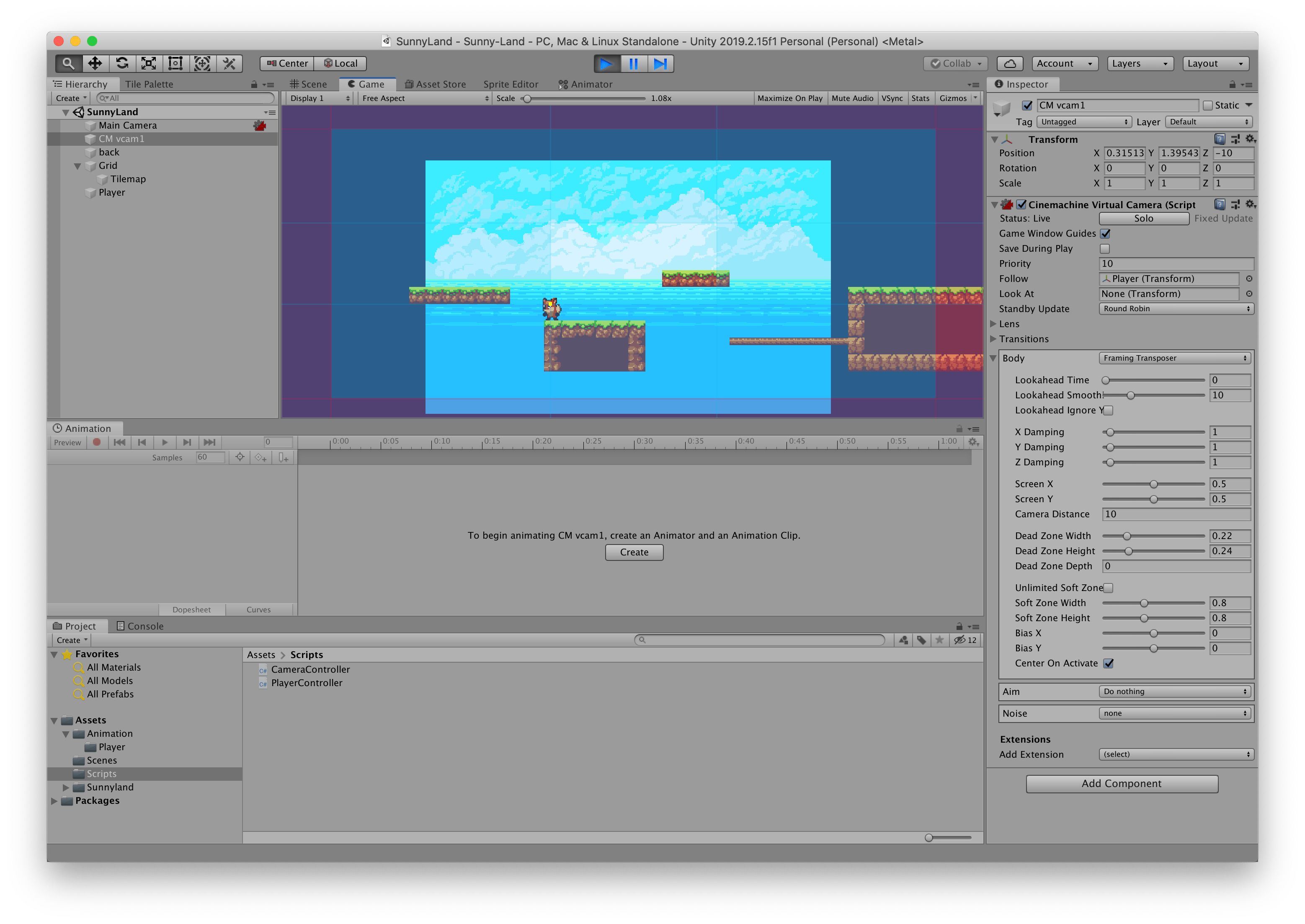
调整背景
- Hierarchy
- Create
- Create Empty:
Background
- Create Empty:
- Create
- Inspector
- Polygon Collider 2D
- IsTrigger:
True
- IsTrigger:
- Polygon Collider 2D
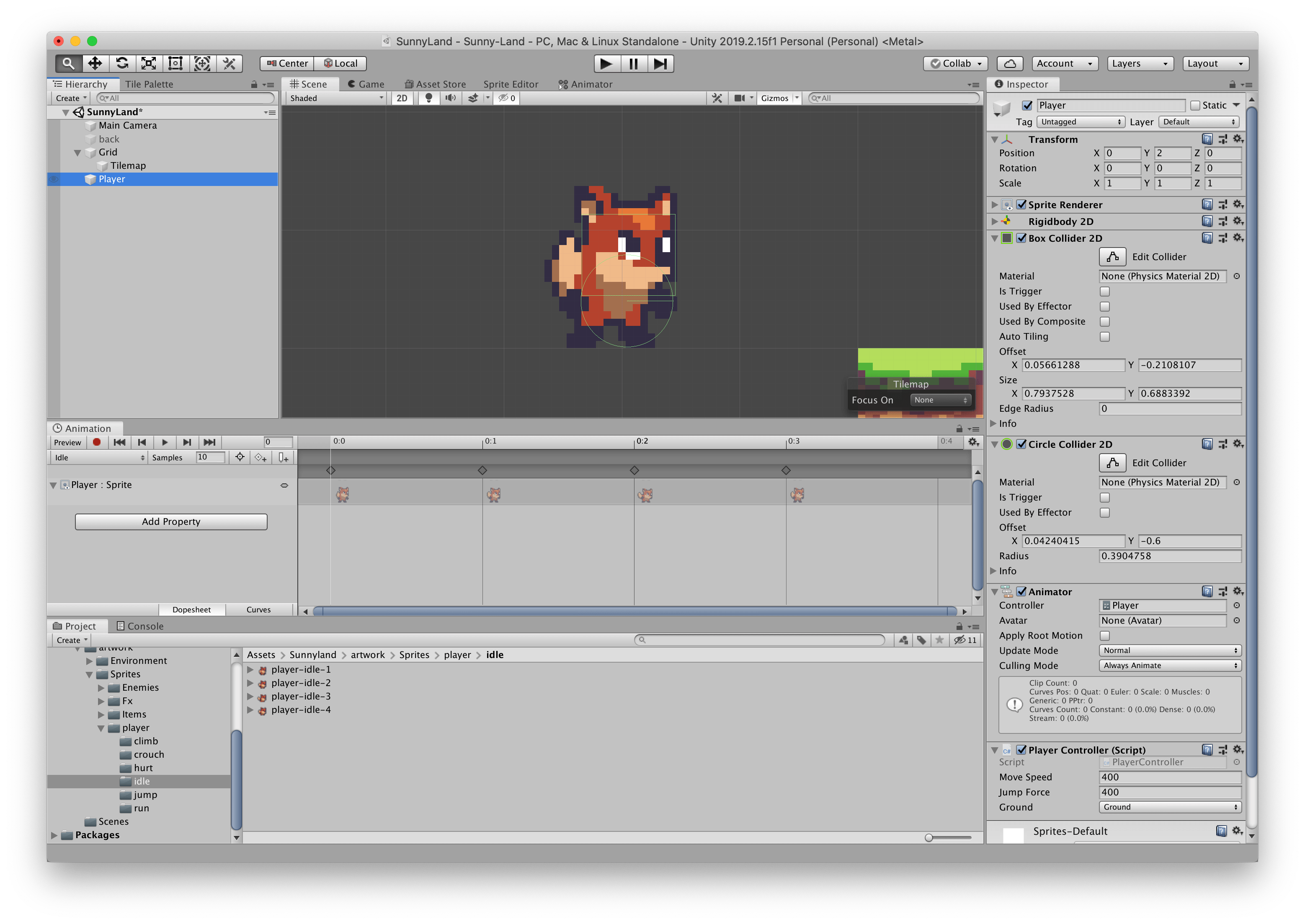
CinemaConfiner / 界限,边界
- Inspector
- Cinemachine Virtual Camera(Script)
- Add Extension
- CinemaConfiner
- Add Extension
- Cinemachine Confiner(Script)
- Bounding Shape 2D:
Background(Polygon Collider 2D)
- Bounding Shape 2D:
- Cinemachine Virtual Camera(Script)
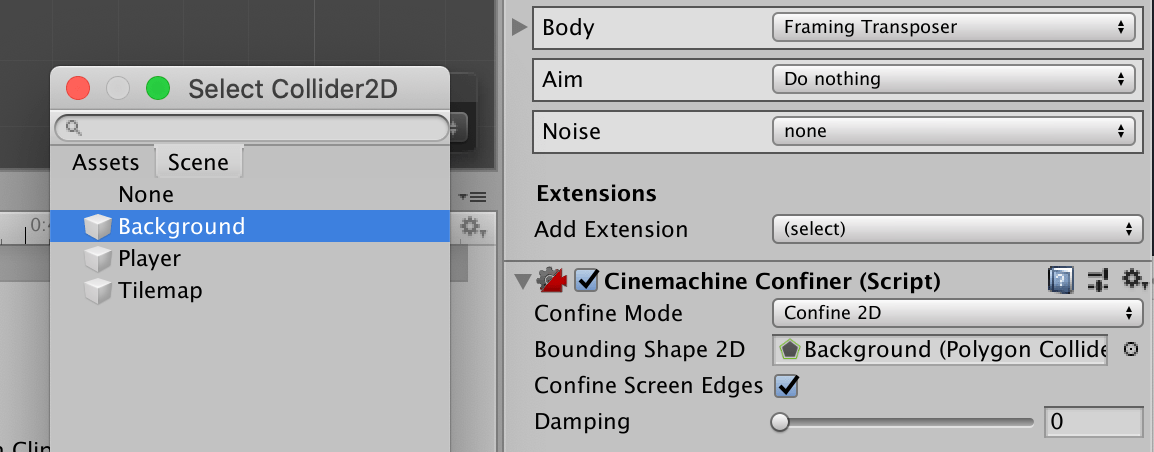
Collections / 收集
cherry & gem: 16 px
- Hierarchy
- Create
- 2D Object
- Sprite:
Cherry
- Sprite:
- 2D Object
- Create
- Inspector
- Tag:
Collection
- Sprite Renderer
- Sprite:
cherry-1
- Sorting Layer:
Foreground
- Sprite:
- Box Collider
- IsTrigger:
true
- IsTrigger:
- Animator
- Controller:
Cherry
<-
- Controller:
- Tag:
- Project
- Assets
- Animation
- Collections
- Animator Controller:
Cherry
- Animator Controller:
- Collections
- Animation
- Assets
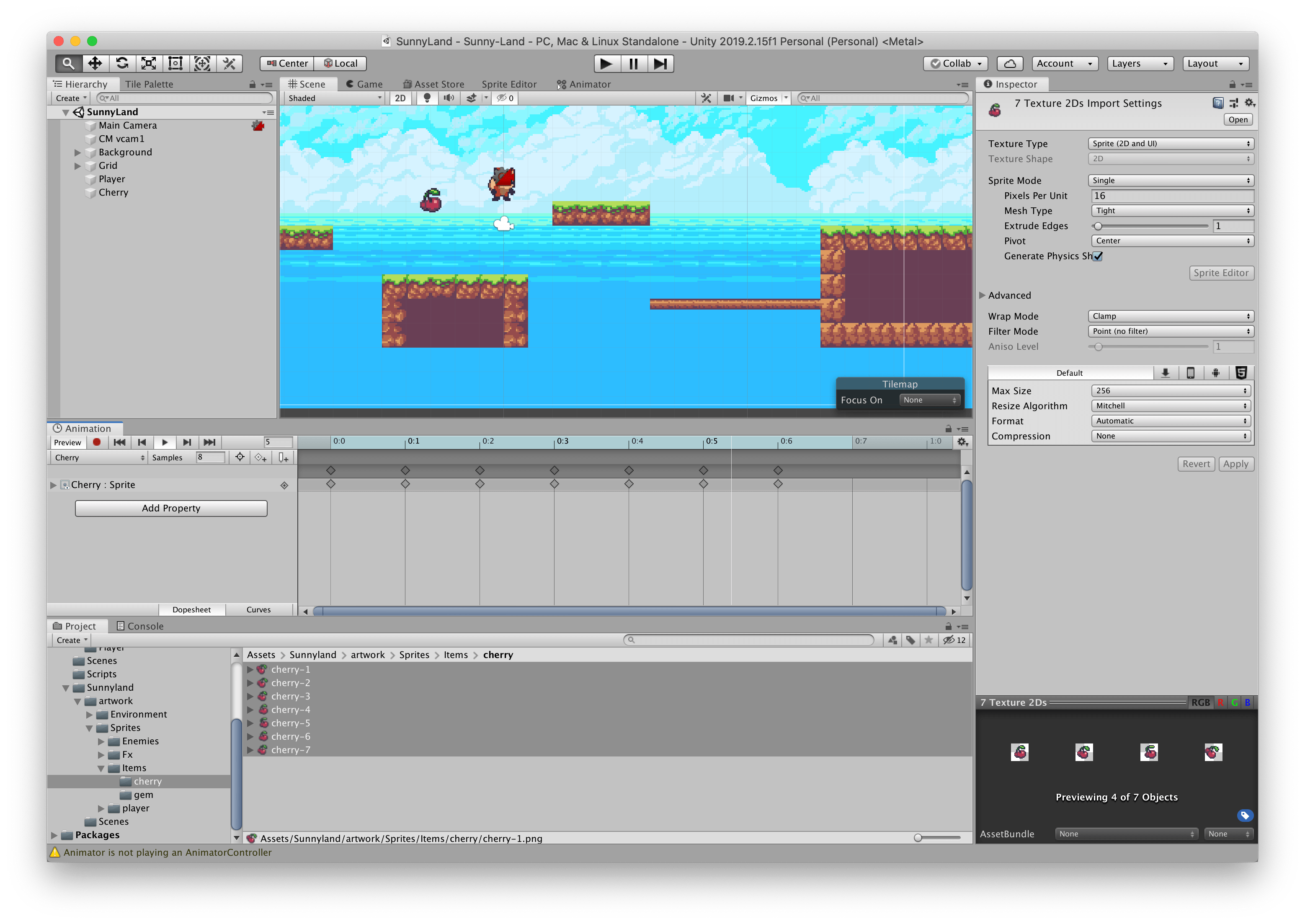
Bug Fix:
- Bug 现象
Player
方向键,粘墙(Tilemap)
- 原因
Player
与Tilemap
之间有磨擦力,不会自动滑落
- 解决方案
Assets
添加Physics Material 2D
,磨擦力(Friction) 置为0
,添加到Player > Box Collider 2D
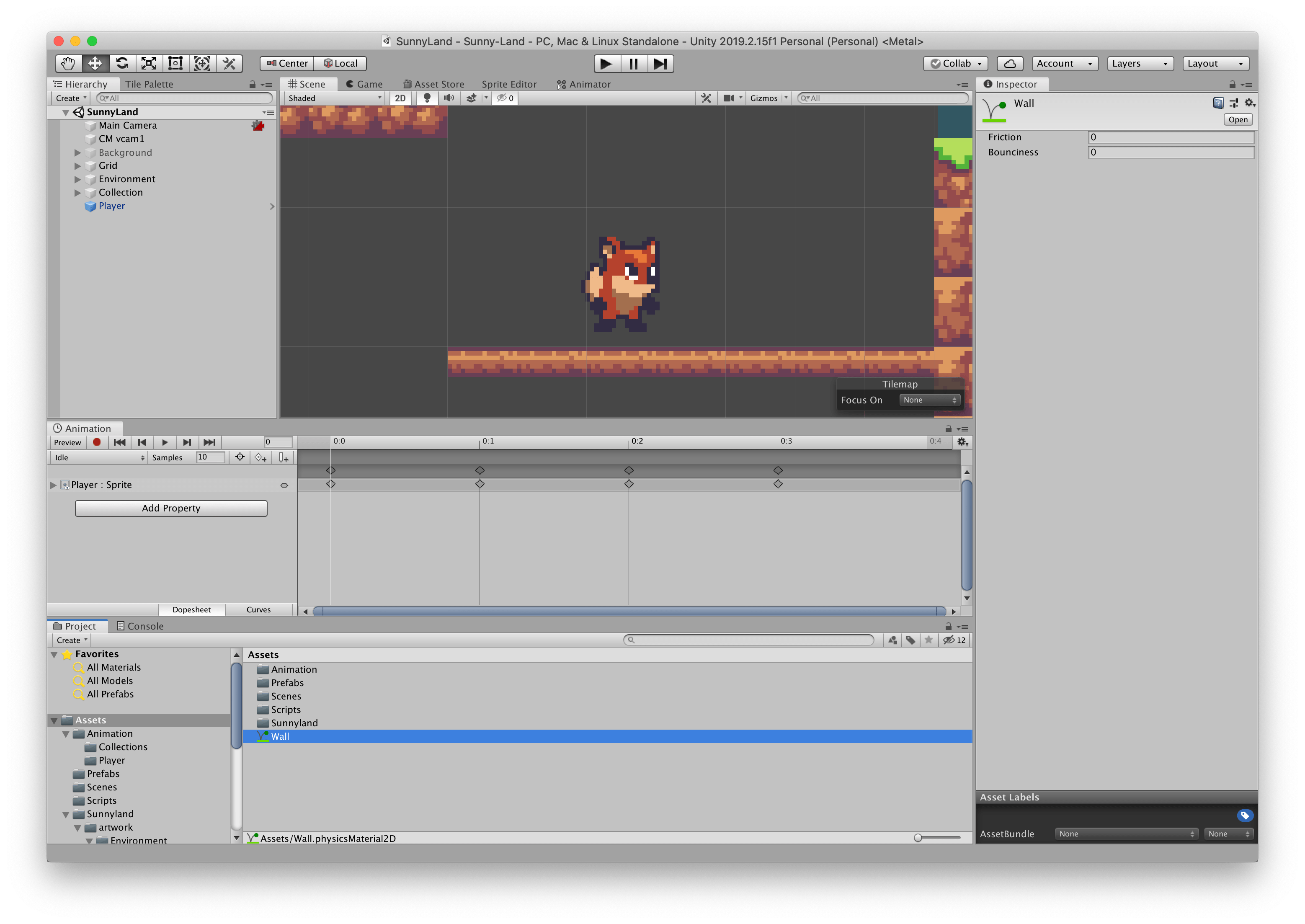
UI
- Hierarchy
- Create
- UI
- Canvas
- UI
- Create
- Canvas
- Create
- UI
- Text:
Cherry
- Text:
Numbers
- Text:
- UI
- Create
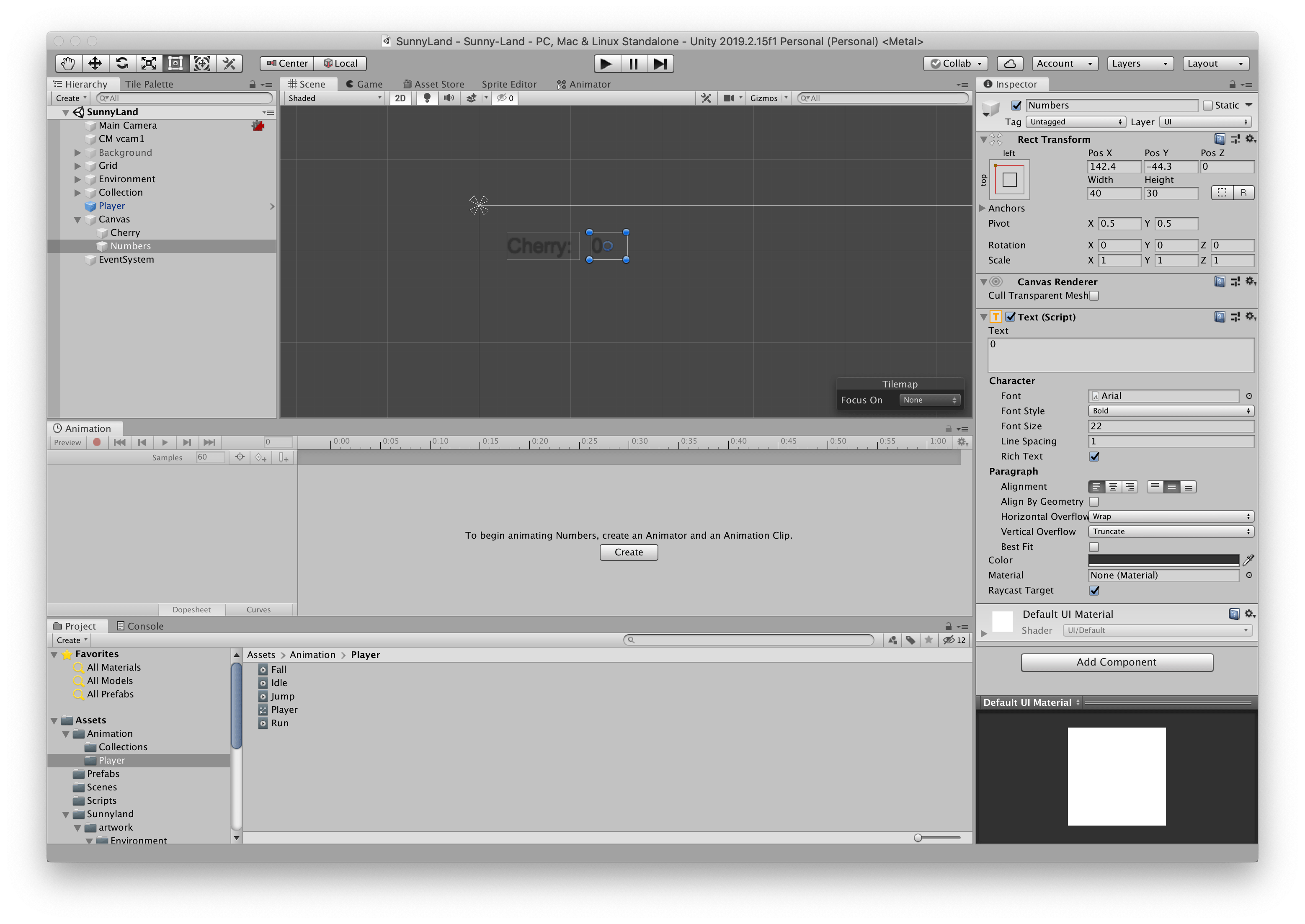
Enemies / 敌人
Frog
- Hierarchy
- Create
- Create Empty:
Enemies
- Create Empty:
- Enemies
- Create
- 2D Object
- Sprite:
Frog
- Sprite:
- 2D Object
- Frog
- Left:
Empty Object
- Right:
Empty Object
- Left:
- Create
- Create
- Inspector
- Tag:
Enemy
- Sprite Renderer
- Sprite:
frog-idle-1
- Sprite:
- Rigidbody 2D
- Constraints
- Freeze Rotation
- Z:
true
- Z:
- Freeze Rotation
- Constraints
- Box Collider 2D
- Edit Collider
- Tag:
创建 Animator Controller: Frog, 拖动到 Frog
创建三种状态
- FrogIdle
- FrogJump
- FrogFall
- Prefabs
- Player
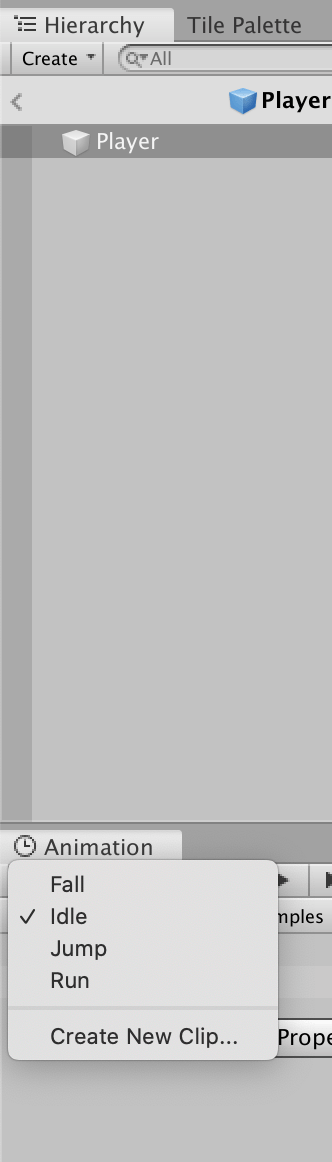
Eagle
从 Assets
中拖动 eagle-attack-1
到 Hierarchy
,重命名为 Eagle
- Hierarchy
- Eagle
- Top:
Empty Object
- Bottom:
Empty Object
- Top:
- Eagle
- Inspector
- Tag:
Enemy
- Sprite Renderer
- Sprite:
eagle-attack-1
- Sprite:
- Rigidbody 2D
- Gravity Scale:
0
# 重力 - Constraints
- Freeze Position
- X:
true
- X:
- Freeze Rotation
- Z:
true
- Z:
- Freeze Position
- Gravity Scale:
- Box Collider 2D
- Edit Collider
- Offset
- X:
-0.4
- Y:
-0.4
- X:
- Radius:
0.5
- Tag:
创建 Animator Controller: Eagle, 拖动到 Eagle
创建一种状态 Eagle
- Prefabs
- Player
Audio / 音效
- Audio Listener: 耳朵
- Audio Source: 扬声器
- Audio Clips: 音乐/声音
Assets
- Sound FX - Retro Pack
- Casual Game BGM
Player BGM / 背景音
- Inspector
- Prefab
- Overrides
- Apply All # 每个
Player Prefab
都包含声音
- Apply All # 每个
- Overrides
- Audio Source
- Loop:
true
- AudioClip:
BGM_01
<-
- Loop:
- Prefab
- Project
- CasualGame
- BGM_01
- CasualGame
Enemy 消灭声音
- Hierarchy
- Enemy
- Frog >
- Enemy
- Inspector
- Audio Source Effects
- Play On Awake:
false
- AudioClip:
hit_04
<-
- Play On Awake:
- Audio Source Effects
- Project
- Zero Rare
- Retro Source
- Hit
- hit_04
- Hit
- Retro Source
- Zero Rare
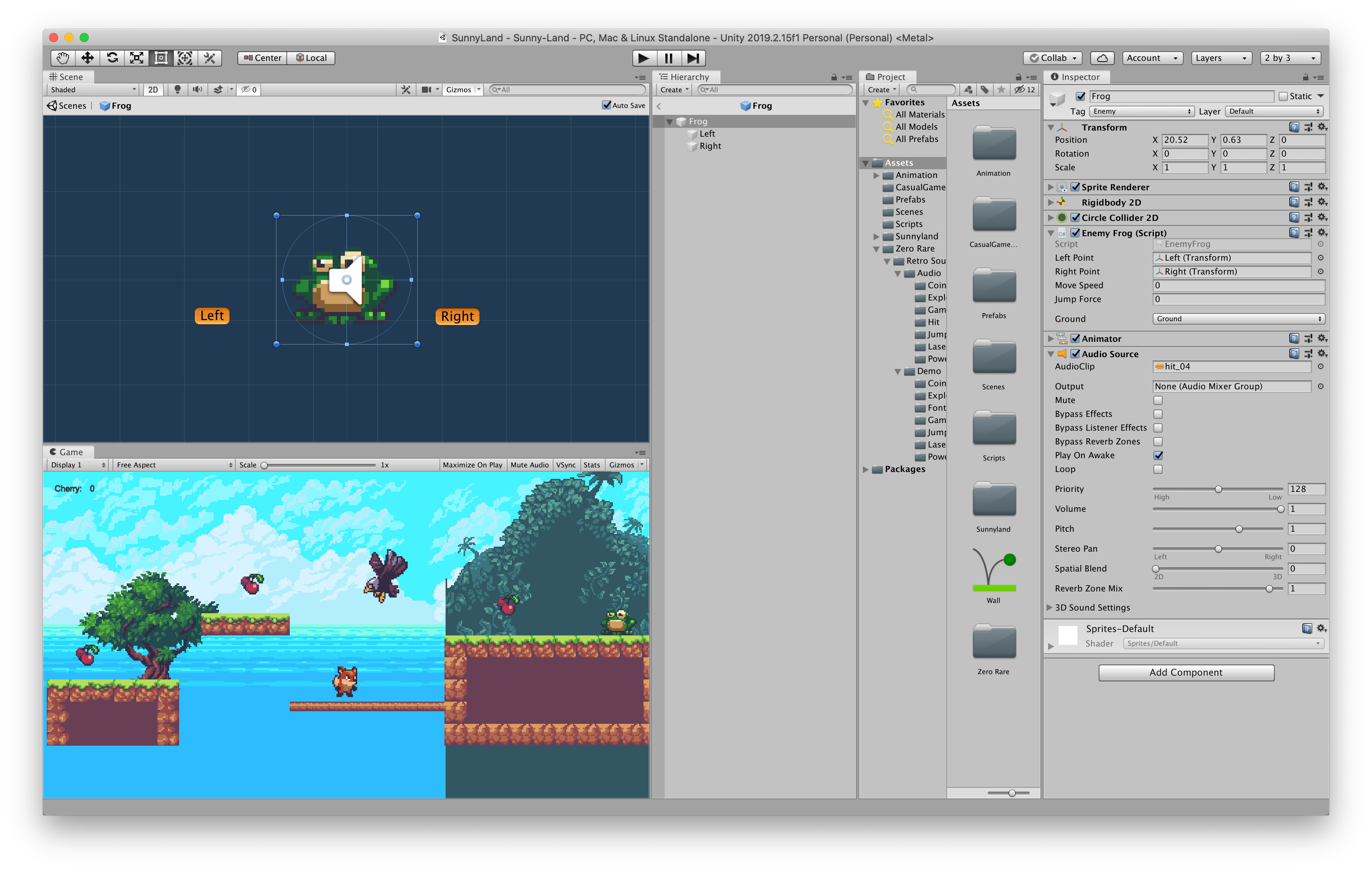
其它声音
- 跳
- 收集
- 。。。
Dialog / 对话框
Assets / 字体
Font: Free Pixel Font - Thaleah
- Hierarchy
- Canves
- Panel:
Dialog
- Text
- Panel:
- Canves
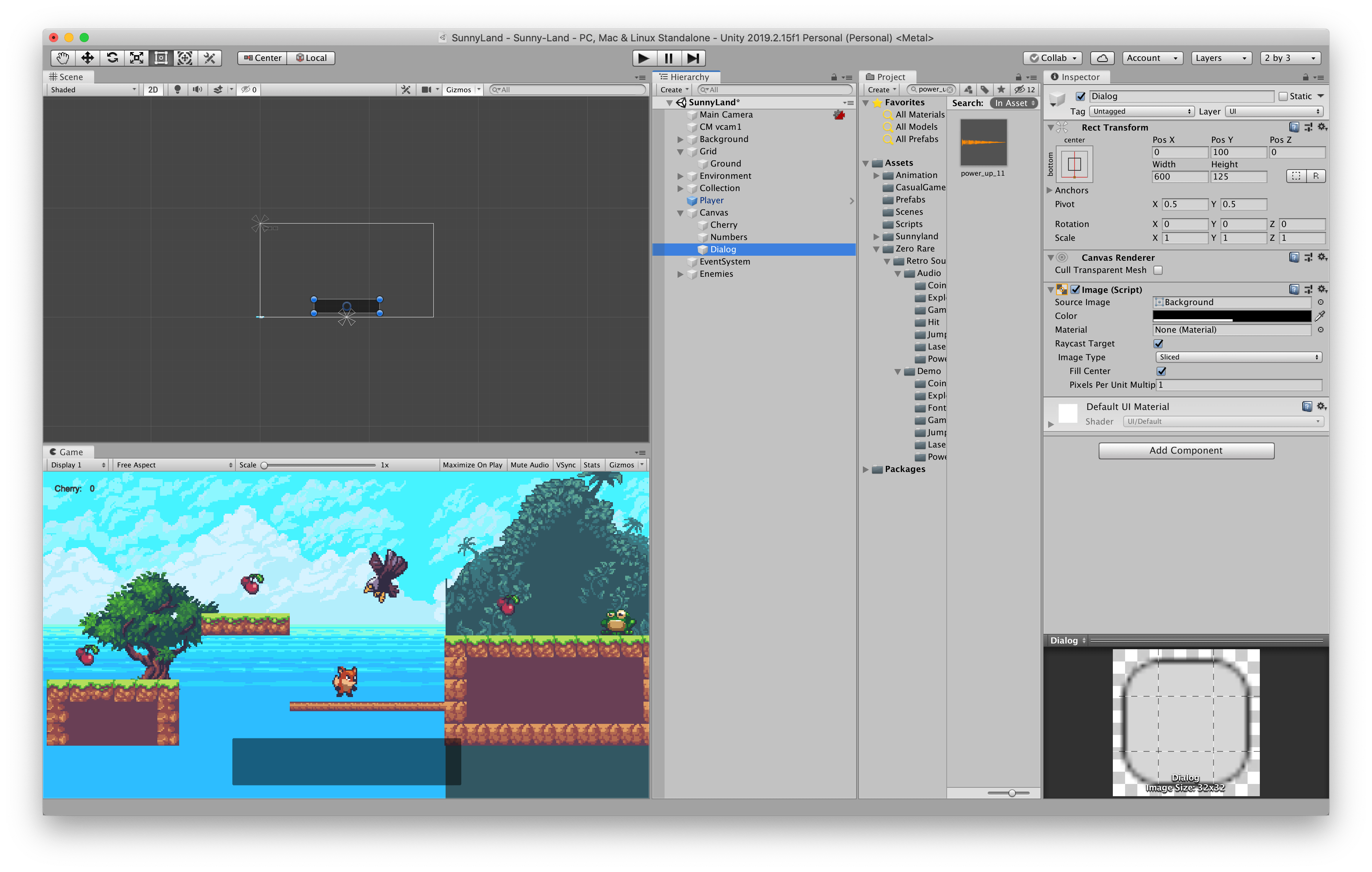
House
- Inspector
- Box Collider 2D
- Edit Collider
- Is Trigger:
true
- Enter Dialog(Script)
- Enter Dialog:
Dialog
- Enter Dialog:
- Box Collider 2D
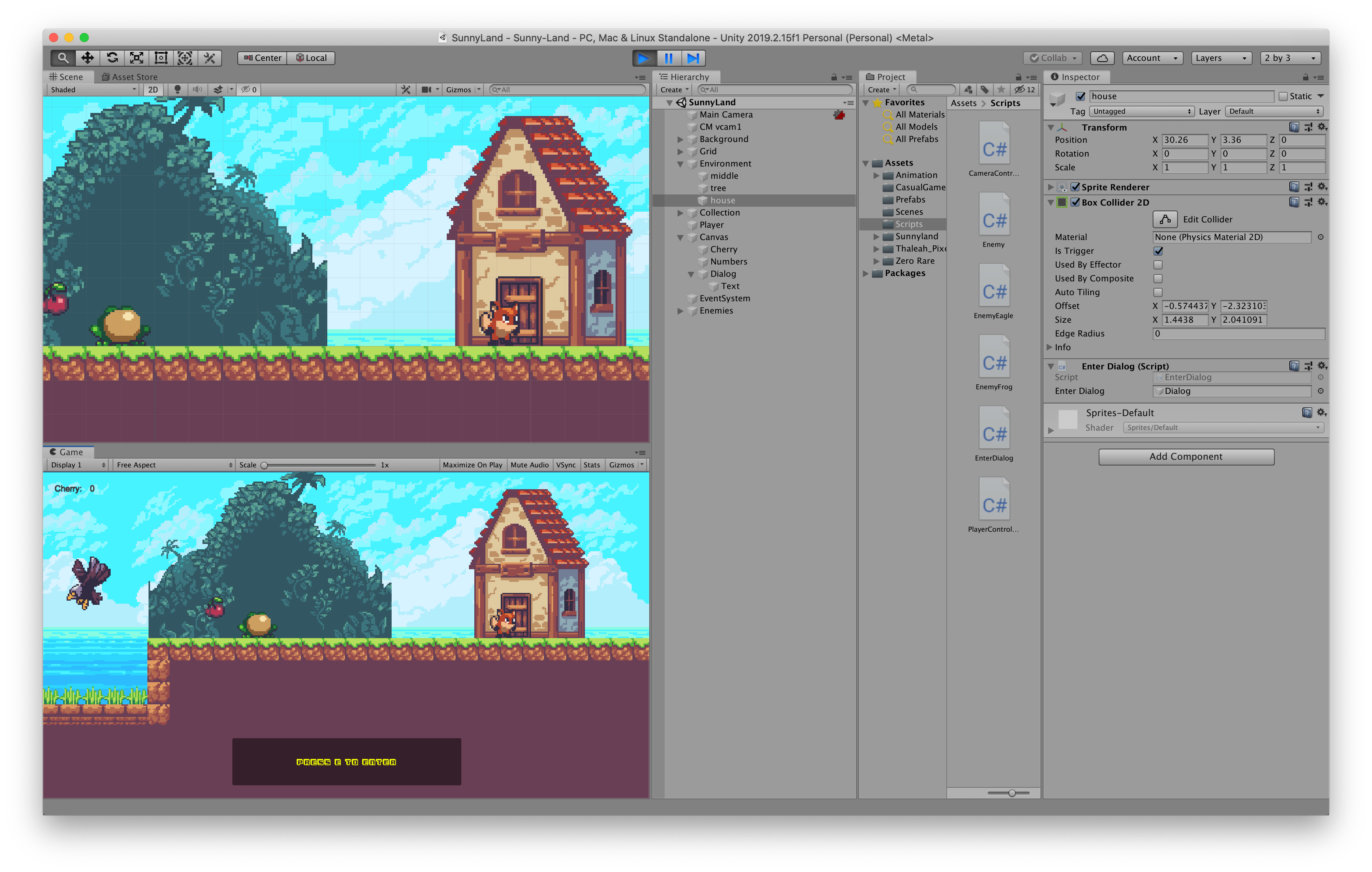
Dialog Animation
- Project
- Animation
- Dialog
- Animation:
Dialog
- Animation:
- Dialog
- Animation
拖动到 UI Dialog
上,自动生成 EnterDialog
Animator
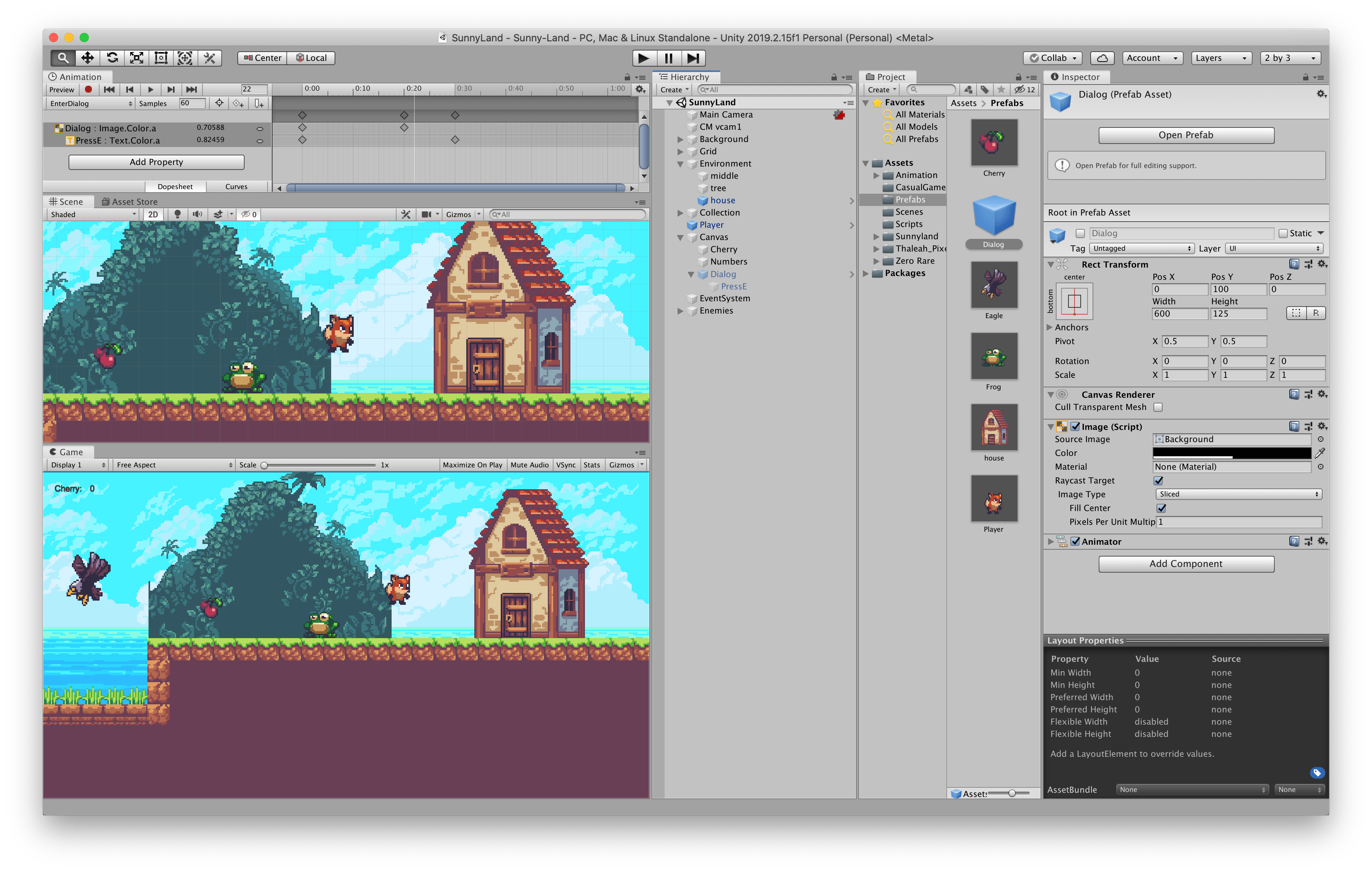
Crouch / 下蹲
- Edit
- Project Settings…
- Input
- Jump
- Duplicate Array Element:
Crouch
- Duplicate Array Element:
- Jump
- Input
- Project Settings…
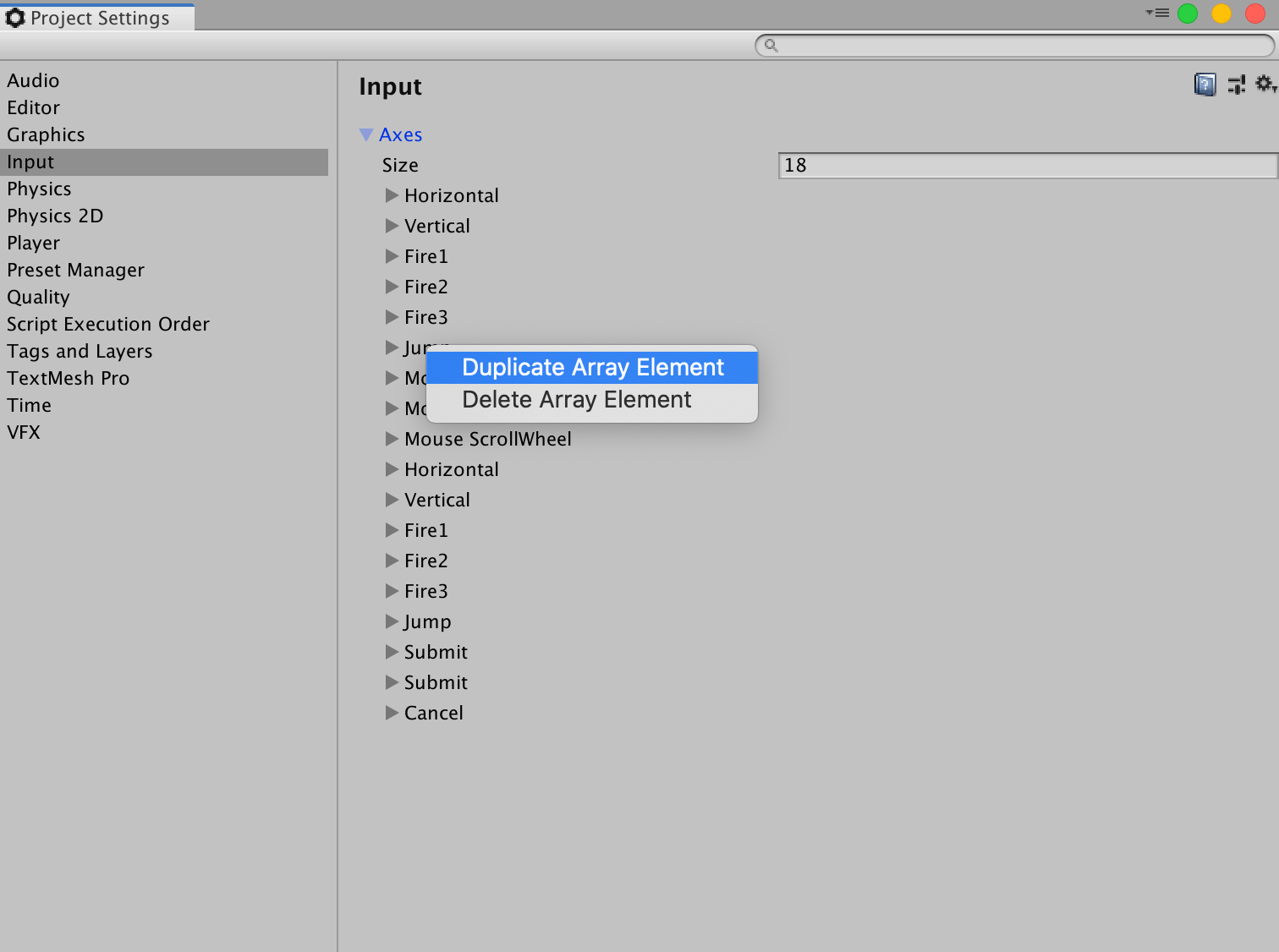
- Crouch
- Positive Button:
s
- Alt Positive Button:
down
- Positive Button:
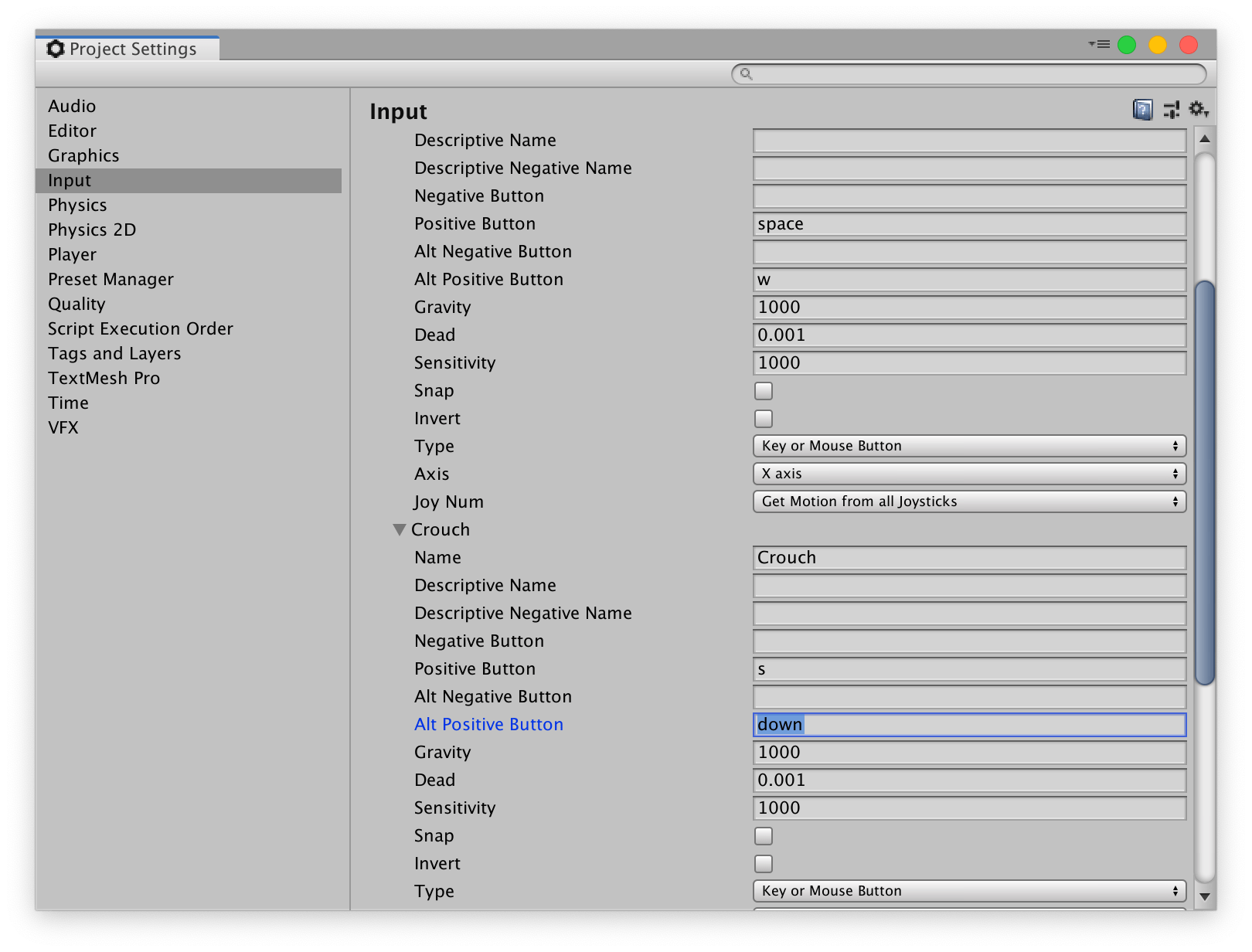
- Project
- Assets
- Animation
- Player
- Animation:
Crouch
- Animation:
- Player
- Animation
- Assets
DeadLine / 死亡
- Hierarchy
- Empty Object:
DeadLine
- Empty Object:
- Inspector
- Tag:
DeadLine
- Transform
- Position
- Y:
-8.3
- Y:
- Position
- Box Collider 2D
- Is Trigger:
True
- Is Trigger:
- Tag:
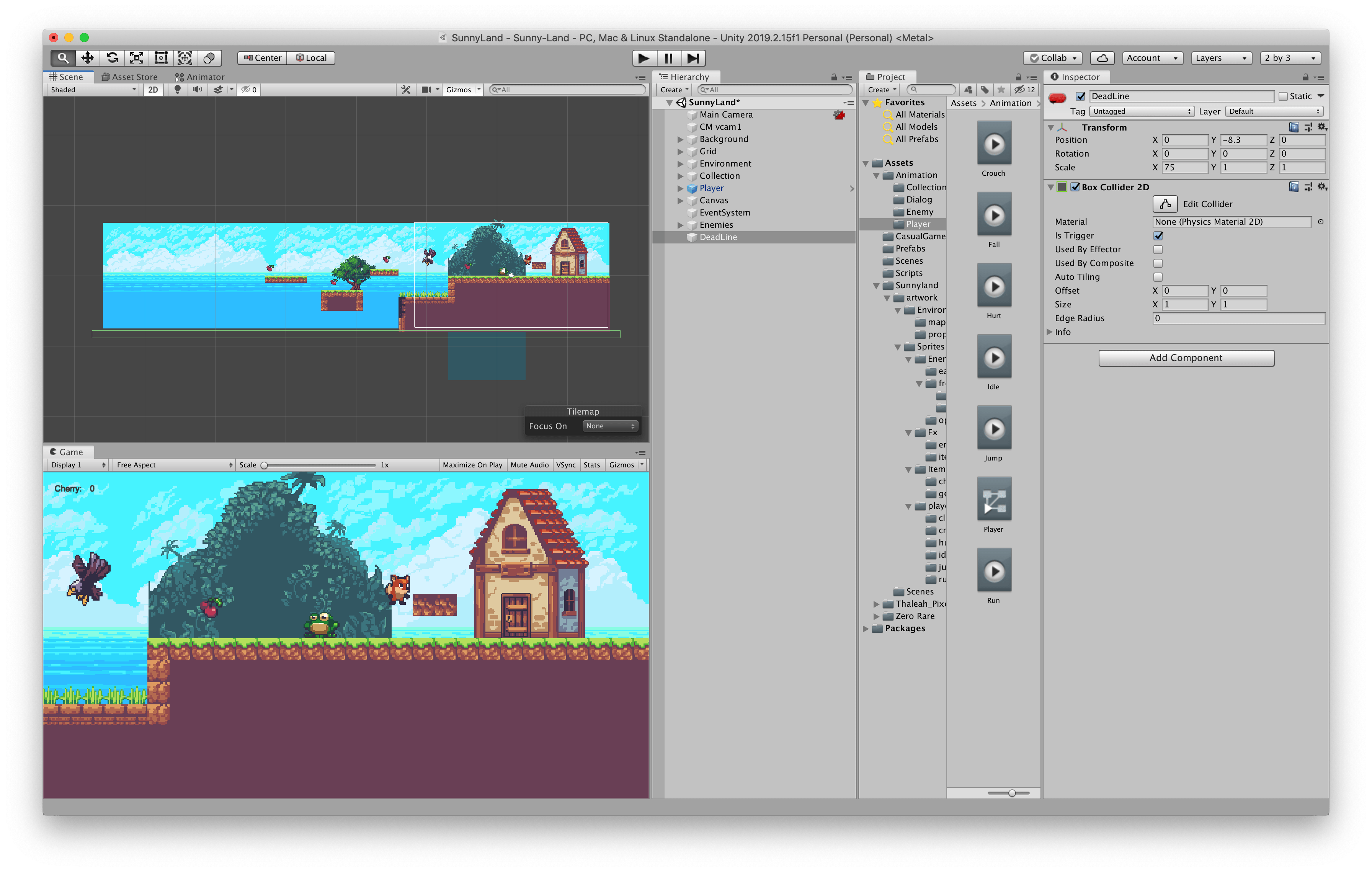
光效
- 素材的 Diffuse 材质
- 法线贴图 Normal map
- 点光源,方向光源
安装 Package
Lightweight RP
- Level_2
- Grid
- Ground
- Grid
- Inspector
- Tilemap Renderer
- Material:
Default-Diffuse
- Material:
- Tilemap Renderer
变暗
导出游戏
- File
- Build Settings (Shift + Cmd + B)
- Player Settings
小结
UI 与 Game 用的是两套不同的坐标。
参考:
下载
游戏
Release: https://github.com/GameDevLog/GameDevLogTemplete/releases